There are a number of ways we can evaluate and read Python code.
Interactively
ipython
setup, which underpins the Jupyter Notebook (inputs/outputs) and provides a sleeker user interface.Interactive programming allows you to dynamically explore code and to make sure your code is doing what you need it to do. It offers a fantastic way to learn the programming environment and problem solve. Moreover, as a data scientist, data structures come in all shapes and sizes and there is not always a "one size fits all" solution. Interactively probing the data in a setup like a Jupyter Notebooks offers us both the flexibility to explore and a way of recording a narrative so that we can remember how we got there.
Script
The advantages of a full scripting language is that we can build larger programs to process our data and then execute those programs in the shell. This offers us a way of streamlining data processing and analysis in important ways.
Somewhere In-Between
Atom
with the Hydrogen
extension. Spyder
is GUI that provides an "RStudio-like" experience when programming in Python.The Jupyter Notebook is an open-source web application that allows you to create and share documents that contain
Uses include: data cleaning and transformation, numerical simulation, statistical modeling, data visualization, machine learning, and much more.
Pros:
R
). This makes Jupyter notebooks particularly friendly when you're first learning PythonAtom
+ Hydrogen
and Spyder
equally useful.Cons:
.ipynb
is really a JSON
file¶At it's core, an Jupyter notebook is a JSON (JavaScript Object Notation) file. Let's see what the notebook that we are currently using looks like:
!cat lecture_03-using-jupyter-notebooks.ipynb
{ "cells": [ { "cell_type": "markdown", "metadata": { "slideshow": { "slide_type": "slide" } }, "source": [ "<h1><center> PPOL564 - Data Science I: Foundations </center><h1>\n", "<h3><center> Lecture 3 <br><br><font color='grey'>Using Jupyter Notebooks</font></center></h3>" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Plan for Today:\n", "\n", "- Ways to use/interact with **Python**\n", "- **Kernels**\n", "- **Using notebooks**\n", "- **Magic Commands**\n", "- Beginning delving into object-oriented programming with Python." ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# Interacting with Python\n", "\n", "There are a number of ways we can evaluate and read Python code.\n", "\n", "**Interactively**\n", "\n", "- From the shell using the **REPL** (\"Read, Evaluate, Print, Loop\")\n", " - Fire up a Python kernel from your terminal\n", " - Alternatively, we can use an `ipython` setup, which underpins the Jupyter Notebook (inputs/outputs) and provides a sleeker user interface.\n", " \n", " \n", "- From a **Jupyter Notebook**\n", "\n", "> Interactive programming allows you to dynamically explore code and to make sure your code is doing what you need it to do. It offers a fantastic way to learn the programming environment and problem solve. Moreover, as a data scientist, data structures come in all shapes and sizes and there is not always a \"one size fits all\" solution. Interactively probing the data in a setup like a Jupyter Notebooks offers us both the flexibility to explore and a way of recording a narrative so that we can remember how we got there. \n", "\n", "**Script**\n", "\n", "- From the shell processing a .py script\n", "\n", "> The advantages of a full scripting language is that we can build larger programs to process our data and then execute those programs in the shell. This offers us a way of streamlining data processing and analysis in important ways. \n", "\n", "**Somewhere _In-Between_**\n", "\n", "- We can leverage both the power of a Jupyter Kernel and the ease of working in a script using `Atom` with the `Hydrogen` extension. \n", "- Likewise, `Spyder` is GUI that provides an \"RStudio-like\" experience when programming in Python." ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# What is a Notebook and why use it?" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "The Jupyter Notebook is an open-source web application that allows you to **create and share documents** that contain \n", "\n", "- live code, \n", "- equations, \n", "- visualizations and \n", "- narrative text. \n", "\n", "Uses include: data cleaning and transformation, numerical simulation, statistical modeling, data visualization, machine learning, and much more. \n", "\n", "**Pros**:\n", "\n", "- Notebooks are **ubiquitous**, \n", "- Reproducible: transmitting and conveying results\n", "- We can **build code interactively** (like we do in `R`). This makes Jupyter notebooks particularly friendly when you're first learning Python\n", " - This also makes `Atom` + `Hydrogen` and `Spyder` equally useful.\n", "- stable\n", "\n", "**Cons**:\n", "\n", "- _Non-linear_: sometimes we can fall out of sequence when writing code. E.g. write code dependencies _after_ we first need to use them.\n", "- There is a process to spinning a Notebook up.\n", "\n", "\n", "### `.ipynb` is really a `JSON` file\n", "At it's core, an Jupyter notebook is a [JSON (JavaScript Object Notation) file](https://en.wikipedia.org/wiki/JSON). Let's see what the notebook that we are currently using looks like:" ] }, { "cell_type": "code", "execution_count": 1, "metadata": { "scrolled": true }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "{\r\n", " \"cells\": [\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {\r\n", " \"slideshow\": {\r\n", " \"slide_type\": \"slide\"\r\n", " }\r\n", " },\r\n", " \"source\": [\r\n", " \"<h1><center> PPOLS564: Foundations of Data Science </center><h1>\\n\",\r\n", " \"<h3><center> Lecture 3 <br><br><font color='grey'>Using Jupyter Notebooks</font></center></h3>\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"## Plan for Today:\\n\",\r\n", " \"\\n\",\r\n", " \"- Ways to use/interact with **Python**\\n\",\r\n", " \"- **Kernels**\\n\",\r\n", " \"- **Using notebooks**\\n\",\r\n", " \"- **Magic Commands**\\n\",\r\n", " \"- Beginning delving into object-oriented programming with Python.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"# Interacting with Python\\n\",\r\n", " \"\\n\",\r\n", " \"There are a number of ways we can evaluate and read Python code.\\n\",\r\n", " \"\\n\",\r\n", " \"**Interactively**\\n\",\r\n", " \"\\n\",\r\n", " \"- From the shell using the **REPL** (\\\"Read, Evaluate, Print, Loop\\\")\\n\",\r\n", " \" - Fire up a Python kernel from your terminal\\n\",\r\n", " \" - Alternatively, we can use an `ipython` setup, which underpins the Jupyter Notebook (inputs/outputs) and provides a sleeker user interface.\\n\",\r\n", " \" \\n\",\r\n", " \" \\n\",\r\n", " \"- From a **Jupyter Notebook**\\n\",\r\n", " \"\\n\",\r\n", " \"> Interactive programming allows you to dynamically explore code and to make sure your code is doing what you need it to do. It offers a fantastic way to learn the programming environment and problem solve. Moreover, as a data scientist, data structures come in all shapes and sizes and there is not always a \\\"one size fits all\\\" solution. Interactively probing the data in a setup like a Jupyter Notebooks offers us both the flexibility to explore and a way of recording a narrative so that we can remember how we got there. \\n\",\r\n", " \"\\n\",\r\n", " \"**Script**\\n\",\r\n", " \"\\n\",\r\n", " \"- From the shell processing a .py script\\n\",\r\n", " \"\\n\",\r\n", " \"> The advantages of a full scripting language is that we can build larger programs to process our data and then execute those programs in the shell. This offers us a way of streamlining data processing and analysis in important ways. \\n\",\r\n", " \"\\n\",\r\n", " \"**Somewhere _In-Between_**\\n\",\r\n", " \"\\n\",\r\n", " \"- We can leverage both the power of a Jupyter Kernel and the ease of working in a script using `Atom` with the `Hydrogen` extension. \\n\",\r\n", " \"- Likewise, `Spyder` is GUI that provides an \\\"RStudio-like\\\" experience when programming in Python.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"# What is a Notebook and why use it?\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"The Jupyter Notebook is an open-source web application that allows you to **create and share documents** that contain \\n\",\r\n", " \"\\n\",\r\n", " \"- live code, \\n\",\r\n", " \"- equations, \\n\",\r\n", " \"- visualizations and \\n\",\r\n", " \"- narrative text. \\n\",\r\n", " \"\\n\",\r\n", " \"Uses include: data cleaning and transformation, numerical simulation, statistical modeling, data visualization, machine learning, and much more. \\n\",\r\n", " \"\\n\",\r\n", " \"**Pros**:\\n\",\r\n", " \"\\n\",\r\n", " \"- Notebooks are **ubiquitous**, \\n\",\r\n", " \"- Reproducible: transmitting and conveying results\\n\",\r\n", " \"- We can **build code interactively** (like we do in `R`). This makes Jupyter notebooks particularly friendly when you're first learning Python\\n\",\r\n", " \" - This also makes `Atom` + `Hydrogen` and `Spyder` equally useful.\\n\",\r\n", " \"- stable\\n\",\r\n", " \"\\n\",\r\n", " \"**Cons**:\\n\",\r\n", " \"\\n\",\r\n", " \"- _Non-linear_: sometimes we can fall out of sequence when writing code. E.g. write code dependencies _after_ we first need to use them.\\n\",\r\n", " \"- There is a process to spinning a Notebook up.\\n\",\r\n", " \"\\n\",\r\n", " \"\\n\",\r\n", " \"### `.ipynb` is really a `JSON` file\\n\",\r\n", " \"At it's core, an Jupyter notebook is a [JSON (JavaScript Object Notation) file](https://en.wikipedia.org/wiki/JSON). Let's see what the notebook that we are currently using looks like:\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 3,\r\n", " \"metadata\": {\r\n", " \"scrolled\": true\r\n", " },\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"{\\r\\n\",\r\n", " \" \\\"cells\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {\\r\\n\",\r\n", " \" \\\"slideshow\\\": {\\r\\n\",\r\n", " \" \\\"slide_type\\\": \\\"slide\\\"\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"<h1><center> PPOLS564: Foundations of Data Science </center><h1>\\\\n\\\",\\r\\n\",\r\n", " \" \\\"<h3><center> Lecture 3 <br><br><font color='grey'>Using Jupyter Notebooks</font></center></h3>\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"## Plan for Today:\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- Ways to use/interact with **Python**\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- **Kernels**\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - Changing Kernels\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- **Usage** \\\\n\\\",\\r\\n\",\r\n", " \" \\\" - **Coding Chunks**\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - Markdown \\\\n\\\",\\r\\n\",\r\n", " \" \\\" - code\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - prints/displays the most recent value in the chunk\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - Using LaTex for mathematical expressions\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - Best Practices\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - **Shortcuts**\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- Setting Working directories \\\\n\\\",\\r\\n\",\r\n", " \" \\\"- **Magic**\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- Reading notebooks from the commandline\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"# Initializing a Notebook\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"# What is a Notebook and Why use it?\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"At it's core, an Jupyter notebook is a [JSON (JavaScript Object Notation) file](https://en.wikipedia.org/wiki/JSON). \\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Let's see what the current \\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": 1,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"name\\\": \\\"stdout\\\",\\r\\n\",\r\n", " \" \\\"output_type\\\": \\\"stream\\\",\\r\\n\",\r\n", " \" \\\"text\\\": [\\r\\n\",\r\n", " \" \\\"{\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cells\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"slideshow\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"slide_type\\\\\\\": \\\\\\\"slide\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"<h1><center> PPOLS564: Foundations of Data Science </center><h1>\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"<h3><center> Lecture 3 <br><br><font color='grey'>Using Jupyter Notebooks</font></center></h3>\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"## Plan for Today:\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- Ways to use/interact with **Python**\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- **Kernels**\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - Changing Kernels\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- **Usage** \\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - **Coding Chunks**\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - Markdown \\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - code\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - prints/displays the most recent value in the chunk\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - Using LaTex for mathematical expressions\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - Best Practices\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - **Shortcuts**\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- Setting Working directories \\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- **Magic**\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- Reading notebooks from the commandline\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"# Initializing a Notebook\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"# What is a Notebook and Why use it?\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"At it's core, an Jupyter notebook is a JSON file. \\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": null,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"%\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"# Kernels\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"A kernel is a computational engine that executes the code contained in a notebook document. A cell (or \\\\\\\\\\\\\\\"Chunk\\\\\\\\\\\\\\\") is a container for text to be displayed in the notebook or code to be executed by the notebook's kernel.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Though we can only have one type of kernel running for any given notebook (we can't change between kernels in the middle of a notebook), we can use jupyter beyond just a python kernel. Here is a [list of all the kernels](https://github.com/jupyter/jupyter/wiki/Jupyter-kernels) that you can use with a jupyter notebook. For example, we can easily employ an [R kernel in a jupyter notebook](https://irkernel.github.io/). This was always the notebooks original intent. Actually, \\\\\\\\\\\\\\\"Jupyter\\\\\\\\\\\\\\\" is a loose acronym meaning Julia, Python and R\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"# Usage\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"## Code Chunks\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"## Shortcuts\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"# Magic\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"# Notebook Extensions\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"We can expand the functionality of Jupyter notebooks through extensions. Extensions allow for use to create and use new features that better customize the notebook's user experience. For example, there are extensions for spell check, a table of contents to ease navigation, and for viewing differences in notebooks when using Version control.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Download python module to install notebook extensions: https://github.com/ipython-contrib/jupyter_contrib_nbextensions\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Using `PyPi` (module manager):\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"```\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pip install jupyter_contrib_nbextensions\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"```\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r", "\r\n", " \" \\\" \\\\\\\"Using `Conda` (Anaconda module manager):\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"```\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"conda install -c conda-forge jupyter_contrib_nbextensions\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"```\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": null,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": []\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": null,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": []\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 2,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"data\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"application/json\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"!\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"HTML\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"SVG\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"bash\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"capture\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"debug\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"file\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"html\\\\\\\": \\\\\\\"DisplayMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"javascript\\\\\\\": \\\\\\\"DisplayMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"js\\\\\\\": \\\\\\\"DisplayMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"latex\\\\\\\": \\\\\\\"DisplayMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"markdown\\\\\\\": \\\\\\\"DisplayMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"perl\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"prun\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pypy\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"python\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"python2\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"python3\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"ruby\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"script\\\\\\\": \\\\\\\"ScriptMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"sh\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"svg\\\\\\\": \\\\\\\"DisplayMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"sx\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"system\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"time\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"timeit\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"writefile\\\\\\\": \\\\\\\"OSMagics\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"line\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"alias\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"alias_magic\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"autocall\\\\\\\": \\\\\\\"AutoMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"automagic\\\\\\\": \\\\\\\"AutoMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"autosave\\\\\\\": \\\\\\\"KernelMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"bookmark\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cat\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cd\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"clear\\\\\\\": \\\\\\\"KernelMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"colors\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"config\\\\\\\": \\\\\\\"ConfigMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"connect_info\\\\\\\": \\\\\\\"KernelMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cp\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"debug\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"dhist\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"dirs\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"doctest_mode\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"ed\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"edit\\\\\\\": \\\\\\\"KernelMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"env\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"gui\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"hist\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"history\\\\\\\": \\\\\\\"HistoryMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"killbgscripts\\\\\\\": \\\\\\\"ScriptMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"ldir\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"less\\\\\\\": \\\\\\\"KernelMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"lf\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"lk\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"ll\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"load\\\\\\\": \\\\\\\"CodeMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"load_ext\\\\\\\": \\\\\\\"ExtensionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"loadpy\\\\\\\": \\\\\\\"CodeMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"logoff\\\\\\\": \\\\\\\"LoggingMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"logon\\\\\\\": \\\\\\\"LoggingMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"logstart\\\\\\\": \\\\\\\"LoggingMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"logstate\\\\\\\": \\\\\\\"LoggingMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"logstop\\\\\\\": \\\\\\\"LoggingMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"ls\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"lsmagic\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"lx\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"macro\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"magic\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"man\\\\\\\": \\\\\\\"KernelMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"matplotlib\\\\\\\": \\\\\\\"PylabMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"mkdir\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"more\\\\\\\": \\\\\\\"KernelMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"mv\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"notebook\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"page\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pastebin\\\\\\\": \\\\\\\"CodeMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pdb\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pdef\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pdoc\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pfile\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pinfo\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pinfo2\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pip\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"popd\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pprint\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"precision\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"profile\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"prun\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"psearch\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"psource\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pushd\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pwd\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pycat\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pylab\\\\\\\": \\\\\\\"PylabMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"qtconsole\\\\\\\": \\\\\\\"KernelMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"quickref\\\\\\\": \\\\\\\"BasicMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"recall\\\\\\\": \\\\\\\"HistoryMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"rehashx\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"reload_ext\\\\\\\": \\\\\\\"ExtensionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"rep\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"rerun\\\\\\\": \\\\\\\"HistoryMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"reset\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"reset_selective\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"rm\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"rmdir\\\\\\\": \\\\\\\"Other\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"run\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"save\\\\\\\": \\\\\\\"CodeMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"sc\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"set_env\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"store\\\\\\\": \\\\\\\"StoreMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"sx\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"system\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"tb\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"time\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"timeit\\\\\\\": \\\\\\\"ExecutionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"unalias\\\\\\\": \\\\\\\"OSMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"unload_ext\\\\\\\": \\\\\\\"ExtensionMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"who\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"who_ls\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"whos\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"xdel\\\\\\\": \\\\\\\"NamespaceMagics\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"xmode\\\\\\\": \\\\\\\"BasicMagics\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"text/plain\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Available line magics:\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"%alias %alias_magic %autocall %automagic %autosave %bookmark %cat %cd %clear %colors %config %connect_info %cp %debug %dhist %dirs %doctest_mode %ed %edit %env %gui %hist %history %killbgscripts %ldir %less %lf %lk %ll %load %load_ext %loadpy %logoff %logon %logstart %logstate %logstop %ls %lsmagic %lx %macro %magic %man %matplotlib %mkdir %more %mv %notebook %page %pastebin %pdb %pdef %pdoc %pfile %pinfo %pinfo2 %popd %pprint %precision %profile %prun %psearch %psource %pushd %pwd %pycat %pylab %qtconsole %quickref %recall %rehashx %reload_ext %rep %rerun %reset %reset_selective %rm %rmdir %run %save %sc %set_env %store %sx %system %tb %time %timeit %unalias %unload_ext %who %who_ls %whos %xdel %xmode\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Available cell magics:\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"%%! %%HTML %%SVG %%bash %%capture %%debug %%file %%html %%javascript %%js %%latex %%markdown %%perl %%prun %%pypy %%python %%python2 %%python3 %%ruby %%script %%sh %%svg %%sx %%system %%time %%timeit %%writefile\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Automagic is ON, % prefix IS NOT needed for line magics.\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 2,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"output_type\\\\\\\": \\\\\\\"execute_result\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"%lsmagic\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Can use **shell** commands within a code chunk\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 30,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"name\\\\\\\": \\\\\\\"stdout\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"output_type\\\\\\\": \\\\\\\"stream\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"text\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\u001b[1m\\\\\\\\u001b[36mCoding-Discussion-01\\\\\\\\u001b[m\\\\\\\\u001b[m \\\\\\\\u001b[1m\\\\\\\\u001b[36mexample-rep1\\\\\\\\u001b[m\\\\\\\\u001b[m text.txt\\\\\\\\r\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\u001b[1m\\\\\\\\u001b[36mRead\\\\\\\\u001b[m\\\\\\\\u001b[m \\\\\\\\u001b[1m\\\\\\\\u001b[36mold\\\\\\\\u001b[m\\\\\\\\u001b[m\\\\\\\\r\\\\\\\\n\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"!ls ~/Desktop\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 6,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"name\\\\\\\": \\\\\\\"stdout\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"output_type\\\\\\\": \\\\\\\"stream\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"text\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Coding-Discussion-01\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"PPOL564-course-materials\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"class-photo.pdf\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"class-photo.png\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"my-project\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"new\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"news-story.txt\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"test-env\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"test-script.py\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"test-test\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"that\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"this\\\\\\\\n\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"%%sh\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"ls ~/Desktop\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"---\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"# Using Python\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"There are a number of ways we can evaluate and read code.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"**Interactively**\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- From the shell using the **REPL** (\\\\\\\\\\\\\\\"Read, Evaluate, Print, Loop\\\\\\\\\\\\\\\")\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - Alternatively, we can use an `ipython` setup\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- From a **Jupyter Notebook**\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - Alternatively, we can activate a Jupyter Kernel in `Atom` using `Hydrogen`\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"> Interactive programming allows you to dynamically explore code and to make sure your code is doing what you need it to do. It offers a fantastic way to learn the programming environment and problem solve. Moreover, as a data scientist, data structures come in all shapes and sizes and there is not always a \\\\\\\\\\\\\\\"one size fits all\\\\\\\\\\\\\\\" solution. Interactively probing the data in a setup like a Jupyter Notebooks offers us both the flexibility to explore and a way of recording a narrative so that we can remember how we got there. \\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"**Script**\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- From the shell processing a .py script\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"> The advantages of a full scripting language is that we can build larger programs to process our data and then execute those programs in the shell. This offers us a way of streamlining data processing and analysis in important ways. \\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"---\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"### Being Pythonic:\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- whitespace is significant\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - Indentations demarcate code blocks. \\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - Four spaces == indentation (PEP8)\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- everything is an object\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- the aim is readable code\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"- Updates to python are recorded in [Python Enhancement Proposals](https://www.python.org/dev/peps/) (or PEPs)\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - When there is a change to python, it is recorded here\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\" - also the python \\\\\\\\\\\\\\\"philosophy\\\\\\\\\\\\\\\" lives here in its suggestions (e.g. PEP8 re: spacing)\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 1,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"code_folding\\\\\\\": []\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"name\\\\\\\": \\\\\\\"stdout\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"output_type\\\\\\\": \\\\\\\"stream\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"text\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"The Zen of Python, by Tim Peters\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Beautiful is better than ugly.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Explicit is better than implicit.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Simple is better than complex.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Complex is better than complicated.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Flat is better than nested.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Sparse is better than dense.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Readability counts.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Special cases aren't special enough to break the rules.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Although practicality beats purity.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Errors should never pass silently.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Unless explicitly silenced.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"In the face of ambiguity, refuse the temptation to guess.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"There should be one-- and preferably only one --obvious way to do it.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Although that way may not be obvious at first unless you're Dutch.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Now is better than never.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Although never is often better than *right* now.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"If the implementation is hard to explain, it's a bad idea.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"If the implementation is easy to explain, it may be a good idea.\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Namespaces are one honking great idea -- let's do more of those!\\\\\\\\n\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"# Python has a sort of philosophy to it. \\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"import this \\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"markdown\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"### Standard Library\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"Python comes with an extensive [standard library](https://docs.python.org/3/library/) and built-in functions.\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\",\\r\\n\",\r\n", " \" \\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 40,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"data\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r", "\r\n", " \" \\\" \\\\\\\"text/plain\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"4.605170185988092\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 40,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"output_type\\\\\\\": \\\\\\\"execute_result\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"import math\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"math.log(100)\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 145,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"data\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"text/plain\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"'That is a dog'\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 145,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"output_type\\\\\\\": \\\\\\\"execute_result\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"import re\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"my_string = \\\\\\\\\\\\\\\"this is a dog\\\\\\\\\\\\\\\"\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"re.sub(\\\\\\\\\\\\\\\"this\\\\\\\\\\\\\\\",\\\\\\\\\\\\\\\"That\\\\\\\\\\\\\\\",my_string)\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"cell_type\\\\\\\": \\\\\\\"code\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 150,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"outputs\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"data\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"text/plain\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"7\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"execution_count\\\\\\\": 150,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {},\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"output_type\\\\\\\": \\\\\\\"execute_result\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"source\\\\\\\": [\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"import random\\\\\\\\n\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"random.randint(1, 10)\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ]\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" ],\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"metadata\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"kernelspec\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"display_name\\\\\\\": \\\\\\\"Python 3\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"language\\\\\\\": \\\\\\\"python\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"name\\\\\\\": \\\\\\\"python3\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"language_info\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"codemirror_mode\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"name\\\\\\\": \\\\\\\"ipython\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"version\\\\\\\": 3\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"file_extension\\\\\\\": \\\\\\\".py\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"mimetype\\\\\\\": \\\\\\\"text/x-python\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"name\\\\\\\": \\\\\\\"python\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"nbconvert_exporter\\\\\\\": \\\\\\\"python\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"pygments_lexer\\\\\\\": \\\\\\\"ipython3\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"version\\\\\\\": \\\\\\\"3.7.0\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"toc\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"base_numbering\\\\\\\": 1,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"nav_menu\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"height\\\\\\\": \\\\\\\"495px\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"width\\\\\\\": \\\\\\\"373.991px\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"number_sections\\\\\\\": false,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"sideBar\\\\\\\": true,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"skip_h1_title\\\\\\\": false,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"title_cell\\\\\\\": \\\\\\\"Table of Contents\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"title_sidebar\\\\\\\": \\\\\\\"Contents\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"toc_cell\\\\\\\": false,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"toc_position\\\\\\\": {\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"height\\\\\\\": \\\\\\\"calc(100% - 180px)\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"left\\\\\\\": \\\\\\\"10px\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"top\\\\\\\": \\\\\\\"150px\\\\\\\",\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"width\\\\\\\": \\\\\\\"165px\\\\\\\"\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"toc_section_display\\\\\\\": true,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"toc_window_display\\\\\\\": false\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" }\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" },\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"nbformat\\\\\\\": 4,\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\" \\\\\\\"nbformat_minor\\\\\\\": 2\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\"}\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\"cat: -h: No such file or directory\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\"cat: 5: No such file or directory\\\\r\\\\n\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"!cat lecture_03-using-jupyter-notebooks.ipynb -h 5\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"# Kernels\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"A kernel is a computational engine that executes the code contained in a notebook document. A cell (or \\\\\\\"Chunk\\\\\\\") is a container for text to be displayed in the notebook or code to be executed by the notebook's kernel.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Though we can only have one type of kernel running for any given notebook (we can't change between kernels in the middle of a notebook), we can use jupyter beyond just a python kernel. Here is a [list of all the kernels](https://github.com/jupyter/jupyter/wiki/Jupyter-kernels) that you can use with a jupyter notebook. For example, we can easily employ an [R kernel in a jupyter notebook](https://irkernel.github.io/). This was always the notebooks original intent. Actually, \\\\\\\"Jupyter\\\\\\\" is a loose acronym meaning Julia, Python and R\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"# Usage\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"## Code Chunks\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"## Shortcuts\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"# Magic\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"# Notebook Extensions\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"We can expand the functionality of Jupyter notebooks through extensions. Extensions allow for use to create and use new features that better customize the notebook's user experience. For example, there are extensions for spell check, a table of contents to ease navigation, and for viewing differences in notebooks when using Version control.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Download python module to install notebook extensions: https://github.com/ipython-contrib/jupyter_contrib_nbextensions\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Using `PyPi` (module manager):\\\\n\\\",\\r\\n\",\r\n", " \" \\\"```\\\\n\\\",\\r\\n\",\r\n", " \" \\\"pip install jupyter_contrib_nbextensions\\\\n\\\",\\r\\n\",\r\n", " \" \\\"```\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Using `Conda` (Anaconda module manager):\\\\n\\\",\\r\\n\",\r\n", " \" \\\"```\\\\n\\\",\\r\\n\",\r\n", " \" \\\"conda install -c conda-forge jupyter_contrib_nbextensions\\\\n\\\",\\r\\n\",\r\n", " \" \\\"```\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": null,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [],\\r\\n\",\r\n", " \" \\\"source\\\": []\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": null,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [],\\r\\n\",\r\n", " \" \\\"source\\\": []\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": 2,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"data\\\": {\\r\\n\",\r\n", " \" \\\"application/json\\\": {\\r\\n\",\r\n", " \" \\\"cell\\\": {\\r\\n\",\r\n", " \" \\\"!\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"HTML\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"SVG\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"bash\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"capture\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"debug\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"file\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"html\\\": \\\"DisplayMagics\\\",\\r\\n\",\r\n", " \" \\\"javascript\\\": \\\"DisplayMagics\\\",\\r\\n\",\r\n", " \" \\\"js\\\": \\\"DisplayMagics\\\",\\r\\n\",\r\n", " \" \\\"latex\\\": \\\"DisplayMagics\\\",\\r\\n\",\r\n", " \" \\\"markdown\\\": \\\"DisplayMagics\\\",\\r\\n\",\r\n", " \" \\\"perl\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"prun\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"pypy\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"python\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"python2\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"python3\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"ruby\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"script\\\": \\\"ScriptMagics\\\",\\r\\n\",\r\n", " \" \\\"sh\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"svg\\\": \\\"DisplayMagics\\\",\\r\\n\",\r\n", " \" \\\"sx\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"system\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"time\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"timeit\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"writefile\\\": \\\"OSMagics\\\"\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"line\\\": {\\r\\n\",\r\n", " \" \\\"alias\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"alias_magic\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"autocall\\\": \\\"AutoMagics\\\",\\r\\n\",\r\n", " \" \\\"automagic\\\": \\\"AutoMagics\\\",\\r\\n\",\r\n", " \" \\\"autosave\\\": \\\"KernelMagics\\\",\\r\\n\",\r\n", " \" \\\"bookmark\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"cat\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"cd\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"clear\\\": \\\"KernelMagics\\\",\\r\\n\",\r\n", " \" \\\"colors\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"config\\\": \\\"ConfigMagics\\\",\\r\\n\",\r\n", " \" \\\"connect_info\\\": \\\"KernelMagics\\\",\\r\\n\",\r\n", " \" \\\"cp\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"debug\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"dhist\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"dirs\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"doctest_mode\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"ed\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"edit\\\": \\\"KernelMagics\\\",\\r\\n\",\r\n", " \" \\\"env\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"gui\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"hist\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"history\\\": \\\"HistoryMagics\\\",\\r\\n\",\r\n", " \" \\\"killbgscripts\\\": \\\"ScriptMagics\\\",\\r\\n\",\r\n", " \" \\\"ldir\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"less\\\": \\\"KernelMagics\\\",\\r\\n\",\r\n", " \" \\\"lf\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"lk\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"ll\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"load\\\": \\\"CodeMagics\\\",\\r\\n\",\r\n", " \" \\\"load_ext\\\": \\\"ExtensionMagics\\\",\\r\\n\",\r\n", " \" \\\"loadpy\\\": \\\"CodeMagics\\\",\\r\\n\",\r\n", " \" \\\"logoff\\\": \\\"LoggingMagics\\\",\\r\\n\",\r\n", " \" \\\"logon\\\": \\\"LoggingMagics\\\",\\r\\n\",\r\n", " \" \\\"logstart\\\": \\\"LoggingMagics\\\",\\r\\n\",\r\n", " \" \\\"logstate\\\": \\\"LoggingMagics\\\",\\r\\n\",\r\n", " \" \\\"logstop\\\": \\\"LoggingMagics\\\",\\r\\n\",\r\n", " \" \\\"ls\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"lsmagic\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"lx\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"macro\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"magic\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"man\\\": \\\"KernelMagics\\\",\\r\\n\",\r\n", " \" \\\"matplotlib\\\": \\\"PylabMagics\\\",\\r\\n\",\r\n", " \" \\\"mkdir\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"more\\\": \\\"KernelMagics\\\",\\r\\n\",\r\n", " \" \\\"mv\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"notebook\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"page\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"pastebin\\\": \\\"CodeMagics\\\",\\r\\n\",\r\n", " \" \\\"pdb\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"pdef\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"pdoc\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"pfile\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"pinfo\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"pinfo2\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"pip\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"popd\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"pprint\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"precision\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"profile\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"prun\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"psearch\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"psource\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"pushd\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"pwd\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"pycat\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"pylab\\\": \\\"PylabMagics\\\",\\r\\n\",\r\n", " \" \\\"qtconsole\\\": \\\"KernelMagics\\\",\\r\\n\",\r\n", " \" \\\"quickref\\\": \\\"BasicMagics\\\",\\r\\n\",\r\n", " \" \\\"recall\\\": \\\"HistoryMagics\\\",\\r\\n\",\r\n", " \" \\\"rehashx\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"reload_ext\\\": \\\"ExtensionMagics\\\",\\r\\n\",\r\n", " \" \\\"rep\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"rerun\\\": \\\"HistoryMagics\\\",\\r\\n\",\r\n", " \" \\\"reset\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"reset_selective\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"rm\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"rmdir\\\": \\\"Other\\\",\\r\\n\",\r\n", " \" \\\"run\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"save\\\": \\\"CodeMagics\\\",\\r\\n\",\r\n", " \" \\\"sc\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"set_env\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"store\\\": \\\"StoreMagics\\\",\\r\\n\",\r\n", " \" \\\"sx\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"system\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"tb\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"time\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"timeit\\\": \\\"ExecutionMagics\\\",\\r\\n\",\r\n", " \" \\\"unalias\\\": \\\"OSMagics\\\",\\r\\n\",\r\n", " \" \\\"unload_ext\\\": \\\"ExtensionMagics\\\",\\r\\n\",\r\n", " \" \\\"who\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"who_ls\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"whos\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"xdel\\\": \\\"NamespaceMagics\\\",\\r\\n\",\r\n", " \" \\\"xmode\\\": \\\"BasicMagics\\\"\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"text/plain\\\": [\\r\\n\",\r\n", " \" \\\"Available line magics:\\\\n\\\",\\r\\n\",\r\n", " \" \\\"%alias %alias_magic %autocall %automagic %autosave %bookmark %cat %cd %clear %colors %config %connect_info %cp %debug %dhist %dirs %doctest_mode %ed %edit %env %gui %hist %history %killbgscripts %ldir %less %lf %lk %ll %load %load_ext %loadpy %logoff %logon %logstart %logstate %logstop %ls %lsmagic %lx %macro %magic %man %matplotlib %mkdir %more %mv %notebook %page %pastebin %pdb %pdef %pdoc %pfile %pinfo %pinfo2 %popd %pprint %precision %profile %prun %psearch %psource %pushd %pwd %pycat %pylab %qtconsole %quickref %recall %rehashx %reload_ext %rep %rerun %reset %reset_selective %rm %rmdir %run %save %sc %set_env %store %sx %system %tb %time %timeit %unalias %unload_ext %who %who_ls %whos %xdel %xmode\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Available cell magics:\\\\n\\\",\\r\\n\",\r\n", " \" \\\"%%! %%HTML %%SVG %%bash %%capture %%debug %%file %%html %%javascript %%js %%latex %%markdown %%perl %%prun %%pypy %%python %%python2 %%python3 %%ruby %%script %%sh %%svg %%sx %%system %%time %%timeit %%writefile\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Automagic is ON, % prefix IS NOT needed for line magics.\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"execution_count\\\": 2,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"output_type\\\": \\\"execute_result\\\"\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"%lsmagic\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"Can use **shell** commands within a code chunk\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": 30,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"name\\\": \\\"stdout\\\",\\r\\n\",\r\n", " \" \\\"output_type\\\": \\\"stream\\\",\\r\\n\",\r\n", " \" \\\"text\\\": [\\r\\n\",\r\n", " \" \\\"\\\\u001b[1m\\\\u001b[36mCoding-Discussion-01\\\\u001b[m\\\\u001b[m \\\\u001b[1m\\\\u001b[36mexample-rep1\\\\u001b[m\\\\u001b[m text.txt\\\\r\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\u001b[1m\\\\u001b[36mRead\\\\u001b[m\\\\u001b[m \\\\u001b[1m\\\\u001b[36mold\\\\u001b[m\\\\u001b[m\\\\r\\\\n\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"!ls ~/Desktop\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": 6,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"name\\\": \\\"stdout\\\",\\r\\n\",\r\n", " \" \\\"output_type\\\": \\\"stream\\\",\\r\\n\",\r\n", " \" \\\"text\\\": [\\r\\n\",\r\n", " \" \\\"Coding-Discussion-01\\\\n\\\",\\r\\n\",\r\n", " \" \\\"PPOL564-course-materials\\\\n\\\",\\r\\n\",\r\n", " \" \\\"class-photo.pdf\\\\n\\\",\\r\\n\",\r\n", " \" \\\"class-photo.png\\\\n\\\",\\r\\n\",\r\n", " \" \\\"my-project\\\\n\\\",\\r\\n\",\r\n", " \" \\\"new\\\\n\\\",\\r\\n\",\r\n", " \" \\\"news-story.txt\\\\n\\\",\\r\\n\",\r\n", " \" \\\"test-env\\\\n\\\",\\r\\n\",\r\n", " \" \\\"test-script.py\\\\n\\\",\\r\\n\",\r\n", " \" \\\"test-test\\\\n\\\",\\r\\n\",\r\n", " \" \\\"that\\\\n\\\",\\r\\n\",\r\n", " \" \\\"this\\\\n\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"%%sh\\\\n\\\",\\r\\n\",\r\n", " \" \\\"ls ~/Desktop\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"---\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"# Using Python\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"There are a number of ways we can evaluate and read code.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"**Interactively**\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- From the shell using the **REPL** (\\\\\\\"Read, Evaluate, Print, Loop\\\\\\\")\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - Alternatively, we can use an `ipython` setup\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- From a **Jupyter Notebook**\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - Alternatively, we can activate a Jupyter Kernel in `Atom` using `Hydrogen`\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"> Interactive programming allows you to dynamically explore code and to make sure your code is doing what you need it to do. It offers a fantastic way to learn the programming environment and problem solve. Moreover, as a data scientist, data structures come in all shapes and sizes and there is not always a \\\\\\\"one size fits all\\\\\\\" solution. Interactively probing the data in a setup like a Jupyter Notebooks offers us both the flexibility to explore and a way of recording a narrative so that we can remember how we got there. \\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"**Script**\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\",\r\n", " \"\\r\\n\",\r\n", " \" \\\"- From the shell processing a .py script\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"> The advantages of a full scripting language is that we can build larger programs to process our data and then execute those programs in the shell. This offers us a way of streamlining data processing and analysis in important ways. \\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"---\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"### Being Pythonic:\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- whitespace is significant\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - Indentations demarcate code blocks. \\\\n\\\",\\r\\n\",\r\n", " \" \\\" - Four spaces == indentation (PEP8)\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- everything is an object\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- the aim is readable code\\\\n\\\",\\r\\n\",\r\n", " \" \\\"- Updates to python are recorded in [Python Enhancement Proposals](https://www.python.org/dev/peps/) (or PEPs)\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - When there is a change to python, it is recorded here\\\\n\\\",\\r\\n\",\r\n", " \" \\\" - also the python \\\\\\\"philosophy\\\\\\\" lives here in its suggestions (e.g. PEP8 re: spacing)\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": 1,\\r\\n\",\r\n", " \" \\\"metadata\\\": {\\r\\n\",\r\n", " \" \\\"code_folding\\\": []\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"outputs\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"name\\\": \\\"stdout\\\",\\r\\n\",\r\n", " \" \\\"output_type\\\": \\\"stream\\\",\\r\\n\",\r\n", " \" \\\"text\\\": [\\r\\n\",\r\n", " \" \\\"The Zen of Python, by Tim Peters\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Beautiful is better than ugly.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Explicit is better than implicit.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Simple is better than complex.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Complex is better than complicated.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Flat is better than nested.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Sparse is better than dense.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Readability counts.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Special cases aren't special enough to break the rules.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Although practicality beats purity.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Errors should never pass silently.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Unless explicitly silenced.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"In the face of ambiguity, refuse the temptation to guess.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"There should be one-- and preferably only one --obvious way to do it.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Although that way may not be obvious at first unless you're Dutch.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Now is better than never.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Although never is often better than *right* now.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"If the implementation is hard to explain, it's a bad idea.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"If the implementation is easy to explain, it may be a good idea.\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Namespaces are one honking great idea -- let's do more of those!\\\\n\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"# Python has a sort of philosophy to it. \\\\n\\\",\\r\\n\",\r\n", " \" \\\"import this \\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"markdown\\\",\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"### Standard Library\\\\n\\\",\\r\\n\",\r\n", " \" \\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"Python comes with an extensive [standard library](https://docs.python.org/3/library/) and built-in functions.\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": 40,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"data\\\": {\\r\\n\",\r\n", " \" \\\"text/plain\\\": [\\r\\n\",\r\n", " \" \\\"4.605170185988092\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"execution_count\\\": 40,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"output_type\\\": \\\"execute_result\\\"\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"import math\\\\n\\\",\\r\\n\",\r\n", " \" \\\"math.log(100)\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": 145,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"data\\\": {\\r\\n\",\r\n", " \" \\\"text/plain\\\": [\\r\",\r\n", " \"\\r\\n\",\r\n", " \" \\\"'That is a dog'\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"execution_count\\\": 145,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"output_type\\\": \\\"execute_result\\\"\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"import re\\\\n\\\",\\r\\n\",\r\n", " \" \\\"my_string = \\\\\\\"this is a dog\\\\\\\"\\\\n\\\",\\r\\n\",\r\n", " \" \\\"re.sub(\\\\\\\"this\\\\\\\",\\\\\\\"That\\\\\\\",my_string)\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"cell_type\\\": \\\"code\\\",\\r\\n\",\r\n", " \" \\\"execution_count\\\": 150,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"outputs\\\": [\\r\\n\",\r\n", " \" {\\r\\n\",\r\n", " \" \\\"data\\\": {\\r\\n\",\r\n", " \" \\\"text/plain\\\": [\\r\\n\",\r\n", " \" \\\"7\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"execution_count\\\": 150,\\r\\n\",\r\n", " \" \\\"metadata\\\": {},\\r\\n\",\r\n", " \" \\\"output_type\\\": \\\"execute_result\\\"\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"source\\\": [\\r\\n\",\r\n", " \" \\\"import random\\\\n\\\",\\r\\n\",\r\n", " \" \\\"random.randint(1, 10)\\\"\\r\\n\",\r\n", " \" ]\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" ],\\r\\n\",\r\n", " \" \\\"metadata\\\": {\\r\\n\",\r\n", " \" \\\"kernelspec\\\": {\\r\\n\",\r\n", " \" \\\"display_name\\\": \\\"Python 3\\\",\\r\\n\",\r\n", " \" \\\"language\\\": \\\"python\\\",\\r\\n\",\r\n", " \" \\\"name\\\": \\\"python3\\\"\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"language_info\\\": {\\r\\n\",\r\n", " \" \\\"codemirror_mode\\\": {\\r\\n\",\r\n", " \" \\\"name\\\": \\\"ipython\\\",\\r\\n\",\r\n", " \" \\\"version\\\": 3\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"file_extension\\\": \\\".py\\\",\\r\\n\",\r\n", " \" \\\"mimetype\\\": \\\"text/x-python\\\",\\r\\n\",\r\n", " \" \\\"name\\\": \\\"python\\\",\\r\\n\",\r\n", " \" \\\"nbconvert_exporter\\\": \\\"python\\\",\\r\\n\",\r\n", " \" \\\"pygments_lexer\\\": \\\"ipython3\\\",\\r\\n\",\r\n", " \" \\\"version\\\": \\\"3.7.0\\\"\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"toc\\\": {\\r\\n\",\r\n", " \" \\\"base_numbering\\\": 1,\\r\\n\",\r\n", " \" \\\"nav_menu\\\": {\\r\\n\",\r\n", " \" \\\"height\\\": \\\"495px\\\",\\r\\n\",\r\n", " \" \\\"width\\\": \\\"373.991px\\\"\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"number_sections\\\": false,\\r\\n\",\r\n", " \" \\\"sideBar\\\": true,\\r\\n\",\r\n", " \" \\\"skip_h1_title\\\": false,\\r\\n\",\r\n", " \" \\\"title_cell\\\": \\\"Table of Contents\\\",\\r\\n\",\r\n", " \" \\\"title_sidebar\\\": \\\"Contents\\\",\\r\\n\",\r\n", " \" \\\"toc_cell\\\": false,\\r\\n\",\r\n", " \" \\\"toc_position\\\": {\\r\\n\",\r\n", " \" \\\"height\\\": \\\"calc(100% - 180px)\\\",\\r\\n\",\r\n", " \" \\\"left\\\": \\\"10px\\\",\\r\\n\",\r\n", " \" \\\"top\\\": \\\"150px\\\",\\r\\n\",\r\n", " \" \\\"width\\\": \\\"165px\\\"\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"toc_section_display\\\": true,\\r\\n\",\r\n", " \" \\\"toc_window_display\\\": false\\r\\n\",\r\n", " \" }\\r\\n\",\r\n", " \" },\\r\\n\",\r\n", " \" \\\"nbformat\\\": 4,\\r\\n\",\r\n", " \" \\\"nbformat_minor\\\": 2\\r\\n\",\r\n", " \"}\\r\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"!cat lecture_03-using-jupyter-notebooks.ipynb\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"# Initializing a Notebook\\n\",\r\n", " \"\\n\",\r\n", " \"There are two primary methods for initializing a notebook. \\n\",\r\n", " \"\\n\",\r\n", " \"1. **Via the command line**\\n\",\r\n", " \" - Go into the working directory containing your `.ipynb` notebook. \\n\",\r\n", " \" - e.g. `cd /Users/me/Desktop/`\\n\",\r\n", " \" - type `jupyter notebook`\\n\",\r\n", " \" - the web application will open up in your default browser. \\n\",\r\n", " \" - from there, click on the notebook and \\\"spin it up\\\". The notebook will then be \\\"running\\\". \\n\",\r\n", " \" - We can close the notebook by clicking on the `Quit` and `Logout` buttons on the page. \\n\",\r\n", " \" - `Quit` == close the local server (i.e. the web application connection)\\n\",\r\n", " \" - `Logout` == shut down the home page of the web application (but keep the server running)\\n\",\r\n", " \" - We can also close the server connection in the console using the combo of `Control-C` in the console. \\n\",\r\n", " \" - We can also relocate the the server (say if we accidentally close the Notebook) by using the local URL pathway provided when the notebook first activates.\\n\",\r\n", " \"\\n\",\r\n", " \"\\n\",\r\n", " \"2. **Via the Anaconda Navigator** (requires you installing an [Anaconda distribution](https://www.anaconda.com/distribution/))\\n\",\r\n", " \"\\n\",\r\n", " \" - Click on the Anaconda Icon\\n\",\r\n", " \" - Click \\\"Launch\\\" on the jupyter notebook icon.\\n\",\r\n", " \" - The web application will immediately fire up (also yielding a console panel much like what we say via the command line approach). \\n\",\r\n", " \" - One issue is that your working directory (i.e. where the notebook thinks you are on your computer) will be where ever Anaconda is stored (for me, it's at the very top of my file directory). Housing your projects here can be suboptimal for a whole range of reasons, so we'll need to **_change the working directory_** to the actual location that we want. \\n\",\r\n", " \" - One benefit of spinning up a Jupyter notebook via the command line is that your working directory will always be where you initialized the notebook. \"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"---\\n\",\r\n", " \"\\n\",\r\n", " \"# Kernels\\n\",\r\n", " \"\\n\",\r\n", " \"A kernel is a computational engine that executes the code contained in a notebook document. A cell (or \\\"Chunk\\\") is a container for text to be displayed in the notebook or code to be executed by the notebook's kernel.\\n\",\r\n", " \"\\n\",\r\n", " \"Though we can only have one type of kernel running for any given notebook (we can't change between kernels in the middle of a notebook), we can use jupyter beyond just a python kernel. Here is a [list of all the kernels](https://github.com/jupyter/jupyter/wiki/Jupyter-kernels) that you can use with a jupyter notebook. For example, we can easily employ an [R kernel in a jupyter notebook](https://irkernel.github.io/). This was always the notebooks original intent. Actually, \\\"Jupyter\\\" is a loose acronym meaning Julia, Python and R\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"---\\n\",\r\n", " \"\\n\",\r\n", " \"# Usage\\n\",\r\n", " \"\\n\",\r\n", " \"\\n\",\r\n", " \"## Code Chunks\\n\",\r\n", " \"\\n\",\r\n", " \"Code chunks are what we use to execute Python (or whatever kernel we have running) code. In addition, we can write prose in a code chunk by altering the metadata regarding how the code should be run.\\n\",\r\n", " \"\\n\",\r\n", " \"There are **two states** of a code chunk:\\n\",\r\n", " \"\\n\",\r\n", " \"- **Edit Mode**: Edit mode is indicated by a <font color =\\\"green\\\">green cell border</font> and a prompt showing in the editor area. When a cell is in edit mode, you can type into the cell, like a normal text editor. Enter edit mode by pressing Enter or using the mouse to click on a cell's editor area.\\n\",\r\n", " \"\\n\",\r\n", " \"\\n\",\r\n", " \"- **Command Mode**: Command mode is indicated by a grey cell border with a <font color = \\\"blue\\\">blue left margin</font>. When you are in command mode, you are able to edit the notebook as a whole, but not type into individual cells. Most importantly, in command mode, the keyboard is mapped to a set of shortcuts that let you perform notebook and cell actions efficiently. For example, if you are in command mode and you press c, you will copy the current cell - no modifier is needed. Don't try to type into a cell in command mode. Enter command mode by pressing `Esc` or using the mouse to click outside a cell's editor area.\\n\",\r\n", " \"\\n\",\r\n", " \"We can **switch between Markdown and Code chunks** either \\n\",\r\n", " \"\\n\",\r\n", " \"- By using the **_drop down menu_** in the tool bar (in either mode)\\n\",\r\n", " \"\\n\",\r\n", " \"\\n\",\r\n", " \"- By using the **_shortcut_**:\\n\",\r\n", " \" - Press `y` when on the cell in Command Mode to switch to a code chunk.\\n\",\r\n", " \" - Press `m` when on the cell in Command Mode to switch to a markdown chunk\\n\",\r\n", " \"\\n\",\r\n", " \"## Executing Code \\n\",\r\n", " \"A code chunk will always reflect the behavior of the kernel that you're using (e.g. a Python code chunk will follow Python coding Syntax). \\n\",\r\n", " \"\\n\",\r\n", " \"**Best Practices**\\n\",\r\n", " \"\\n\",\r\n", " \"- Break code chunks up! \\n\",\r\n", " \"- Every code chunk should render some output (the aim is to be able to read what we were doing without needing to fire the notebook back up)\\n\",\r\n", " \"- Use spaces. Keep the chunk readable. Less is more.\\n\",\r\n", " \"\\n\",\r\n", " \"## Using Markdown\\n\",\r\n", " \"The Markdown chunks will use the [Markdown](https://www.markdownguide.org/) and will allow for writing mathematical equations using LaTex. \"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"# Header 1\\n\",\r\n", " \"## Header 2\\n\",\r\n", " \"### Header 3\\n\",\r\n", " \"#### Header 4\\n\",\r\n", " \"\\n\",\r\n", " \"Bullet points \\n\",\r\n", " \"\\n\",\r\n", " \"- _Italics_ or *Italics*\\n\",\r\n", " \"- **Bold**\\n\",\r\n", " \"- **_Both_**\\n\",\r\n", " \"\\n\",\r\n", " \"Enumerated Lists\\n\",\r\n", " \" \\n\",\r\n", " \"1. _Italics_ or *Italics*\\n\",\r\n", " \"2. **Bold**\\n\",\r\n", " \"3. **_Both_**\\n\",\r\n", " \"\\n\",\r\n", " \"We can <u>underline</u> using html tags. Likewise, we can change <font color =\\\"darkred\\\">font colors</font>,\\n\",\r\n", " \"\\n\",\r\n", " \"<center> center </center>\\n\",\r\n", " \"\\n\",\r\n", " \"And include [hyperlinks](https://en.wikipedia.org/wiki/Grape)\\n\",\r\n", " \"\\n\",\r\n", " \"Write math inline with `$$`. For example, $y_i = \\\\beta_0 + \\\\beta_1 x_i + \\\\epsilon$\\n\",\r\n", " \"\\n\",\r\n", " \"Or stand alone,\\n\",\r\n", " \"\\n\",\r\n", " \"$$pr(y_i=1) = \\\\frac{1}{1+e^{\\\\beta_0 + \\\\beta_1 x_i + \\\\epsilon}}$$\\n\",\r", "\r\n", " \"\\n\",\r\n", " \"We can embed images. \\n\",\r\n", " \"\\n\",\r\n", " \"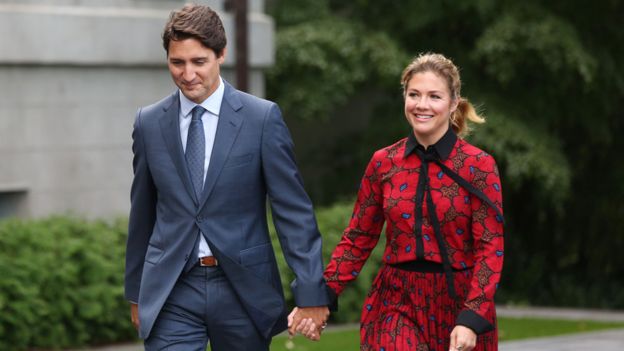\\n\",\r\n", " \"\\n\",\r\n", " \"And videos!\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 19,\r\n", " \"metadata\": {\r\n", " \"scrolled\": true\r\n", " },\r\n", " \"outputs\": [\r\n", " {\r\n", " \"data\": {\r\n", " \"text/html\": [\r\n", " \"<iframe width=\\\"900\\\" height=\\\"300\\\" frameborder=\\\"0\\\" src=\\\"https://www.bbc.com/news/av/embed/p07n10lt/49656611\\\"></iframe>\"\r\n", " ],\r\n", " \"text/plain\": [\r\n", " \"<IPython.core.display.HTML object>\"\r\n", " ]\r\n", " },\r\n", " \"metadata\": {},\r\n", " \"output_type\": \"display_data\"\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%%HTML\\n\",\r\n", " \"<iframe width=\\\"900\\\" height=\\\"300\\\" frameborder=\\\"0\\\" src=\\\"https://www.bbc.com/news/av/embed/p07n10lt/49656611\\\"></iframe>\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"## Using the Shell (Command Line)\\n\",\r\n", " \"\\n\",\r\n", " \"As we saw in lab, we can use the command line from directly inside a notebook by preceding all shell code with a `!`. This allows use to really streamline our coding process. \"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 13,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"/Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03\\r\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"!pwd # Current working directory\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 15,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"On branch master\\r\\n\",\r\n", " \"Your branch is up to date with 'origin/master'.\\r\\n\",\r\n", " \"\\r\\n\",\r\n", " \"Changes not staged for commit:\\r\\n\",\r\n", " \" (use \\\"git add <file>...\\\" to update what will be committed)\\r\\n\",\r\n", " \" (use \\\"git checkout -- <file>...\\\" to discard changes in working directory)\\r\\n\",\r\n", " \"\\r\\n\",\r\n", " \"\\t\\u001b[31mmodified: ../lecture_02/lecture_02_version-control.ipynb\\u001b[m\\r\\n\",\r\n", " \"\\r\\n\",\r\n", " \"Untracked files:\\r\\n\",\r\n", " \" (use \\\"git add <file>...\\\" to include in what will be committed)\\r\\n\",\r\n", " \"\\r\\n\",\r\n", " \"\\t\\u001b[31m./\\u001b[m\\r\\n\",\r\n", " \"\\r\\n\",\r\n", " \"no changes added to commit (use \\\"git add\\\" and/or \\\"git commit -a\\\")\\r\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"!git status #checking our git status (anything we need to commit?)\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 17,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"lecture_03-using-jupyter-notebooks.ipynb\\r\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"!ls #list off all the files in the current working directory.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"Also note that we can use inline magic to use shell commands.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 38,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"1\\n\",\r\n", " \"2\\n\",\r\n", " \"3\\n\",\r\n", " \"4\\n\",\r\n", " \"5\\n\",\r\n", " \"6\\n\",\r\n", " \"7\\n\",\r\n", " \"8\\n\",\r\n", " \"9\\n\",\r\n", " \"10\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%%sh \\n\",\r\n", " \"for i in {1..10}\\n\",\r\n", " \"do\\n\",\r\n", " \" echo $i\\n\",\r\n", " \"done\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"## Shortcuts\\n\",\r\n", " \"\\n\",\r\n", " \"As with most user interfaces, Jupyter Notebooks have developed their own way of doing things. Thus there are a number of useful shortcuts that you can employ to help perform useful tasks. \\n\",\r\n", " \"\\n\",\r\n", " \"We can access a full (searchable) list of keyboard shortcuts by pressing `p` when in Command Mode, or by clicking the keyboard icon in the tools.\\n\",\r\n", " \"\\n\",\r\n", " \"Important ones while in Command Mode:\\n\",\r\n", " \"\\n\",\r\n", " \"- `a`: create a new code chunk _above_ the current one.\\n\",\r\n", " \"- `b`: create a new code chunk _below_ the current one.\\n\",\r\n", " \"- `ii`: interrupt the kernel (really useful when some code is running too long or you've accidentally initiated an infinite loop!\\n\",\r\n", " \"- `y`: code mode\\n\",\r\n", " \"- `m`: markdown mode\\n\",\r\n", " \"- `shift` + `m`: merge cells (when more than one cell is highlighted)\\n\",\r\n", " \"\\n\",\r\n", " \"Important ones while in Edit Mode:\\n\",\r\n", " \"\\n\",\r\n", " \"- `shit` + `ctrl` + `minus`: split cell\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"---\\n\",\r\n", " \"\\n\",\r\n", " \"# Magic Commands\\n\",\r\n", " \"\\n\",\r\n", " \"Magic commands, and are prefixed by the `%` character. These magic commands are designed to succinctly solve various common problems in standard data analysis. \\n\",\r\n", " \"\\n\",\r\n", " \"Magic commands come in two flavors: \\n\",\r\n", " \"\\n\",\r\n", " \"- **line magics**, which are denoted by a _single_ `%` prefix and operate on a single line of input, \\n\",\r\n", " \"- **cell magics**, which are denoted by a _double_ `%%` prefix and operate on multiple lines of input. \\n\",\r\n", " \"\\n\",\r\n", " \"List off all the available magic commands.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 43,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " \"data\": {\r\n", " \"application/json\": {\r\n", " \"cell\": {\r\n", " \"!\": \"OSMagics\",\r\n", " \"HTML\": \"Other\",\r\n", " \"SVG\": \"Other\",\r\n", " \"bash\": \"Other\",\r\n", " \"capture\": \"ExecutionMagics\",\r\n", " \"debug\": \"ExecutionMagics\",\r\n", " \"file\": \"Other\",\r\n", " \"html\": \"DisplayMagics\",\r\n", " \"javascript\": \"DisplayMagics\",\r\n", " \"js\": \"DisplayMagics\",\r\n", " \"latex\": \"DisplayMagics\",\r\n", " \"markdown\": \"DisplayMagics\",\r\n", " \"perl\": \"Other\",\r\n", " \"prun\": \"ExecutionMagics\",\r\n", " \"pypy\": \"Other\",\r\n", " \"python\": \"Other\",\r\n", " \"python2\": \"Other\",\r\n", " \"python3\": \"Other\",\r\n", " \"ruby\": \"Other\",\r\n", " \"script\": \"ScriptMagics\",\r\n", " \"sh\": \"Other\",\r\n", " \"svg\": \"DisplayMagics\",\r\n", " \"sx\": \"OSMagics\",\r\n", " \"system\": \"OSMagics\",\r\n", " \"time\": \"ExecutionMagics\",\r\n", " \"timeit\": \"ExecutionMagics\",\r\n", " \"writefile\": \"OSMagics\"\r\n", " },\r\n", " \"line\": {\r\n", " \"alias\": \"OSMagics\",\r\n", " \"alias_magic\": \"BasicMagics\",\r\n", " \"autocall\": \"AutoMagics\",\r\n", " \"automagic\": \"AutoMagics\",\r\n", " \"autosave\": \"KernelMagics\",\r\n", " \"bookmark\": \"OSMagics\",\r\n", " \"cat\": \"Other\",\r\n", " \"cd\": \"OSMagics\",\r\n", " \"clear\": \"KernelMagics\",\r\n", " \"colors\": \"BasicMagics\",\r\n", " \"config\": \"ConfigMagics\",\r\n", " \"connect_info\": \"KernelMagics\",\r\n", " \"cp\": \"Other\",\r\n", " \"debug\": \"ExecutionMagics\",\r\n", " \"dhist\": \"OSMagics\",\r\n", " \"dirs\": \"OSMagics\",\r\n", " \"doctest_mode\": \"BasicMagics\",\r\n", " \"ed\": \"Other\",\r\n", " \"edit\": \"KernelMagics\",\r\n", " \"env\": \"OSMagics\",\r\n", " \"gui\": \"BasicMagics\",\r\n", " \"hist\": \"Other\",\r\n", " \"history\": \"HistoryMagics\",\r\n", " \"killbgscripts\": \"ScriptMagics\",\r\n", " \"ldir\": \"Other\",\r\n", " \"less\": \"KernelMagics\",\r\n", " \"lf\": \"Other\",\r\n", " \"lk\": \"Other\",\r\n", " \"ll\": \"Other\",\r\n", " \"load\": \"CodeMagics\",\r\n", " \"load_ext\": \"ExtensionMagics\",\r\n", " \"loadpy\": \"CodeMagics\",\r\n", " \"logoff\": \"LoggingMagics\",\r\n", " \"logon\": \"LoggingMagics\",\r\n", " \"logstart\": \"LoggingMagics\",\r\n", " \"logstate\": \"LoggingMagics\",\r\n", " \"logstop\": \"LoggingMagics\",\r\n", " \"ls\": \"Other\",\r\n", " \"lsmagic\": \"BasicMagics\",\r\n", " \"lx\": \"Other\",\r\n", " \"macro\": \"ExecutionMagics\",\r\n", " \"magic\": \"BasicMagics\",\r\n", " \"man\": \"KernelMagics\",\r\n", " \"matplotlib\": \"PylabMagics\",\r\n", " \"mkdir\": \"Other\",\r\n", " \"more\": \"KernelMagics\",\r\n", " \"mv\": \"Other\",\r\n", " \"notebook\": \"BasicMagics\",\r\n", " \"page\": \"BasicMagics\",\r\n", " \"pastebin\": \"CodeMagics\",\r\n", " \"pdb\": \"ExecutionMagics\",\r\n", " \"pdef\": \"NamespaceMagics\",\r\n", " \"pdoc\": \"NamespaceMagics\",\r\n", " \"pfile\": \"NamespaceMagics\",\r\n", " \"pinfo\": \"NamespaceMagics\",\r\n", " \"pinfo2\": \"NamespaceMagics\",\r\n", " \"pip\": \"BasicMagics\",\r\n", " \"popd\": \"OSMagics\",\r\n", " \"pprint\": \"BasicMagics\",\r\n", " \"precision\": \"BasicMagics\",\r\n", " \"profile\": \"BasicMagics\",\r\n", " \"prun\": \"ExecutionMagics\",\r\n", " \"psearch\": \"NamespaceMagics\",\r\n", " \"psource\": \"NamespaceMagics\",\r\n", " \"pushd\": \"OSMagics\",\r\n", " \"pwd\": \"OSMagics\",\r\n", " \"pycat\": \"OSMagics\",\r\n", " \"pylab\": \"PylabMagics\",\r\n", " \"qtconsole\": \"KernelMagics\",\r\n", " \"quickref\": \"BasicMagics\",\r\n", " \"recall\": \"HistoryMagics\",\r\n", " \"rehashx\": \"OSMagics\",\r\n", " \"reload_ext\": \"ExtensionMagics\",\r\n", " \"rep\": \"Other\",\r\n", " \"rerun\": \"HistoryMagics\",\r\n", " \"reset\": \"NamespaceMagics\",\r\n", " \"reset_selective\": \"NamespaceMagics\",\r\n", " \"rm\": \"Other\",\r\n", " \"rmdir\": \"Other\",\r\n", " \"run\": \"ExecutionMagics\",\r\n", " \"save\": \"CodeMagics\",\r\n", " \"sc\": \"OSMagics\",\r\n", " \"set_env\": \"OSMagics\",\r\n", " \"store\": \"StoreMagics\",\r\n", " \"sx\": \"OSMagics\",\r\n", " \"system\": \"OSMagics\",\r\n", " \"tb\": \"ExecutionMagics\",\r\n", " \"time\": \"ExecutionMagics\",\r\n", " \"timeit\": \"ExecutionMagics\",\r\n", " \"unalias\": \"OSMagics\",\r\n", " \"unload_ext\": \"ExtensionMagics\",\r\n", " \"who\": \"NamespaceMagics\",\r\n", " \"who_ls\": \"NamespaceMagics\",\r\n", " \"whos\": \"NamespaceMagics\",\r\n", " \"xdel\": \"NamespaceMagics\",\r\n", " \"xmode\": \"BasicMagics\"\r\n", " }\r\n", " },\r\n", " \"text/plain\": [\r\n", " \"Available line magics:\\n\",\r\n", " \"%alias %alias_magic %autocall %automagic %autosave %bookmark %cat %cd %clear %colors %config %connect_info %cp %debug %dhist %dirs %doctest_mode %ed %edit %env %gui %hist %history %killbgscripts %ldir %less %lf %lk %ll %load %load_ext %loadpy %logoff %logon %logstart %logstate %logstop %ls %lsmagic %lx %macro %magic %man %matplotlib %mkdir %more %mv %notebook %page %pastebin %pdb %pdef %pdoc %pfile %pinfo %pinfo2 %popd %pprint %precision %profile %prun %psearch %psource %pushd %pwd %pycat %pylab %qtconsole %quickref %recall %rehashx %reload_ext %rep %rerun %reset %reset_selective %rm %rmdir %run %save %sc %set_env %store %sx %system %tb %time %timeit %unalias %unload_ext %who %who_ls %whos %xdel %xmode\\n\",\r\n", " \"\\n\",\r\n", " \"Available cell magics:\\n\",\r\n", " \"%%! %%HTML %%SVG %%bash %%capture %%debug %%file %%html %%javascript %%js %%latex %%markdown %%perl %%prun %%pypy %%python %%python2 %%python3 %%ruby %%script %%sh %%svg %%sx %%system %%time %%timeit %%writefile\\n\",\r\n", " \"\\n\",\r\n", " \"Automagic is ON, % prefix IS NOT needed for line magics.\"\r\n", " ]\r\n", " },\r\n", " \"execution_count\": 43,\r\n", " \"metadata\": {},\r\n", " \"output_type\": \"execute_result\"\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%lsmagic\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"Or consult the quick reference sheet of all available magic\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 66,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"%quickref\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"## Useful Magic\\n\",\r\n", " \"\\n\",\r\n", " \"Here are some useful magic commands that come in handy as you're working with code.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Bookmarking\\n\",\r\n", " \"\\\"Come back here later\\\"\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 46,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"%bookmark Home\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"See below\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Changing working directories \"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 47,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"/Users/ericdunford/Desktop\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%cd ~/Desktop\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 48,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"data\": {\r\n", " \"text/plain\": [\r\n", " \"'/Users/ericdunford/Desktop'\"\r\n", " ]\r\n", " },\r\n", " \"execution_count\": 48,\r\n", " \"metadata\": {},\r\n", " \"output_type\": \"execute_result\"\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%pwd\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"Using the bookmark to return to where we were...\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 51,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"(bookmark:Home) -> /Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03\\n\",\r\n", " \"/Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%cd -b Home\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 52,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"data\": {\r\n", " \"text/plain\": [\r\n", " \"'/Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03'\"\r\n", " ]\r\n", " },\r\n", " \"execution_count\": 52,\r\n", " \"metadata\": {},\r\n", " \"output_type\": \"execute_result\"\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%pwd\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Writing code to files\\n\",\r\n", " \"\\n\",\r\n", " \"Extremely useful when we develop some functionality that we'd like to utilize later on.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 60,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"Writing my_fib_func.py\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%%writefile my_fib_func.py\\n\",\r\n", " \"def fib(n):\\n\",\r\n", " \" '''Fibonacci Sequence'''\\n\",\r\n", " \" x = [0]*n\\n\",\r\n", " \" for i in range(n):\\n\",\r\n", " \" if i == 0:\\n\",\r\n", " \" x[i] = 0\\n\",\r\n", " \" elif i == 1:\\n\",\r\n", " \" x[i] = 1\\n\",\r\n", " \" else:\\n\",\r\n", " \" x[i] = x[i-2] + x[i-1]\\n\",\r\n", " \" return x\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 64,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"lecture_03-using-jupyter-notebooks.ipynb\\r\\n\",\r\n", " \"my_fib_func.py\\r\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%ls # list files ( see our function)\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Reading in files\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": null,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"%load my_fib_func.py\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Run an external file as a program\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 81,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"%run my_fib_func.py\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Timing Code\\n\",\r\n", " \"\\n\",\r\n", " \"How fast does what we wrote run?\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 70,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"CPU times: user 3 µs, sys: 0 ns, total: 3 µs\\n\",\r\n", " \"Wall time: 7.15 µs\\n\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"data\": {\r\n", " \"text/plain\": [\r\n", " \"[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]\"\r\n", " ]\r\n", " },\r\n", " \"execution_count\": 70,\r\n", " \"metadata\": {},\r\n", " \"output_type\": \"execute_result\"\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%time \\n\",\r\n", " \"fib(10)\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"How long does many runs take (statistical sample)?\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 69,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"2.84 µs ± 30.6 ns per loop (mean ± std. dev. of 7 runs, 100000 loops each)\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"%%timeit\\n\",\r\n", " \"fib(10)\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Look up object names in the name space\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 84,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"main_dat = [1,2,3,4]\\n\",\r\n", " \"main_key = [\\\"a\\\",\\\"b\\\"]\\n\",\r\n", " \"x = 5\\n\",\r\n", " \"y = 6\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 87,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"%psearch main*\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"Whenever you encounter an error or exception, just open a new notebook cell, type `%debug` and run the cell. This will open a command line where you can test your code and inspect all variables right up to the line that threw the error. Type `n` and hit Enter to run the next line of code (The `->` arrow shows you the current position). Use `c` to continue until the next breakpoint. `q` quits the debugger and code execution.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Asking for help\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 90,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"%%timeit?\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"---\\n\",\r\n", " \"\\n\",\r\n", " \"# Notebook Extensions\\n\",\r\n", " \"\\n\",\r\n", " \"We can expand the functionality of Jupyter notebooks through extensions. Extensions allow for use to create and use new features that better customize the notebook's user experience. For example, there are extensions for spell check, a table of contents to ease navigation, run code in parallel, and for viewing differences in notebooks when using Version control.\\n\",\r\n", " \"\\n\",\r\n", " \"Download python module to install notebook extensions: https://github.com/ipython-contrib/jupyter_contrib_nbextensions\\n\",\r\n", " \"\\n\",\r\n", " \"\\n\",\r\n", " \"Using `PyPi` (module manager):\\n\",\r\n", " \"```\\n\",\r\n", " \"pip install jupyter_nbextensions_configurator jupyter_contrib_nbextensions\\n\",\r\n", " \"jupyter contrib nbextension install --user\\n\",\r\n", " \"jupyter nbextensions_configurator enable --user\\n\",\r\n", " \"```\\n\",\r\n", " \"\\n\",\r\n", " \"Using `Conda` (Anaconda module manager):\\n\",\r\n", " \"```\\n\",\r\n", " \"conda install -c conda-forge jupyter_contrib_nbextensions\\n\",\r\n", " \"jupyter contrib nbextension install --user\\n\",\r\n", " \"jupyter nbextensions_configurator enable --user\\n\",\r\n", " \"```\\n\",\r\n", " \"\\n\",\r\n", " \"Extensions can be activated most easily on the home screen when you first activate your Jupyter notebook.\\n\",\r\n", " \"\\n\",\r\n", " \"\\n\",\r\n", " \"## Useful Extensions\\n\",\r\n", " \"\\n\",\r\n", " \"- **Collapsible headings**: allows you to collapse some parts of the notebooks.\\n\",\r\n", " \"- **Notify**: sends a notification when the notebook becomes idle (for long running tasks)\\n\",\r\n", " \"- **Code folding**: folds function, loops, and indented code chunks (makes things tidy)\\n\",\r\n", " \"- **nbdime**: provides tools for git differencing and merging of Jupyter Notebooks.\\n\",\r\n", " \" - Requires installation: `pip install nbdime`\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"---\\n\",\r\n", " \"\\n\",\r\n", " \"# Being Pythonic\\n\",\r\n", " \"\\n\",\r\n", " \"- whitespace is significant\\n\",\r\n", " \" - Indentations demarcate code blocks. \\n\",\r\n", " \" - Four spaces == indentation (PEP8)\\n\",\r\n", " \"- everything is an object\\n\",\r\n", " \"- the aim is readable code\\n\",\r\n", " \"- Updates to python are recorded in [Python Enhancement Proposals](https://www.python.org/dev/peps/) (or PEPs)\\n\",\r\n", " \" - When there is a change to python, it is recorded here\\n\",\r\n", " \" - also the python \\\"philosophy\\\" lives here in its suggestions (e.g. PEP8 re: spacing)\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 4,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"The Zen of Python, by Tim Peters\\n\",\r\n", " \"\\n\",\r\n", " \"Beautiful is better than ugly.\\n\",\r\n", " \"Explicit is better than implicit.\\n\",\r\n", " \"Simple is better than complex.\\n\",\r\n", " \"Complex is better than complicated.\\n\",\r\n", " \"Flat is better than nested.\\n\",\r\n", " \"Sparse is better than dense.\\n\",\r\n", " \"Readability counts.\\n\",\r\n", " \"Special cases aren't special enough to break the rules.\\n\",\r\n", " \"Although practicality beats purity.\\n\",\r\n", " \"Errors should never pass silently.\\n\",\r\n", " \"Unless explicitly silenced.\\n\",\r\n", " \"In the face of ambiguity, refuse the temptation to guess.\\n\",\r\n", " \"There should be one-- and preferably only one --obvious way to do it.\\n\",\r\n", " \"Although that way may not be obvious at first unless you're Dutch.\\n\",\r\n", " \"Now is better than never.\\n\",\r\n", " \"Although never is often better than *right* now.\\n\",\r\n", " \"If the implementation is hard to explain, it's a bad idea.\\n\",\r\n", " \"If the implementation is easy to explain, it may be a good idea.\\n\",\r\n", " \"Namespaces are one honking great idea -- let's do more of those!\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"# Python has a sort of philosophy to it. \\n\",\r\n", " \"import this \"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Standard Library\\n\",\r\n", " \"\\n\",\r\n", " \"Python comes with an extensive [standard library](https://docs.python.org/3/library/) and built-in functions.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 40,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"data\": {\r\n", " \"text/plain\": [\r\n", " \"4.605170185988092\"\r\n", " ]\r\n", " },\r\n", " \"execution_count\": 40,\r\n", " \"metadata\": {},\r\n", " \"output_type\": \"execute_result\"\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"import math\\n\",\r\n", " \"math.log(100)\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 145,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"data\": {\r\n", " \"text/plain\": [\r\n", " \"'That is a dog'\"\r\n", " ]\r\n", " },\r\n", " \"execution_count\": 145,\r\n", " \"metadata\": {},\r\n", " \"output_type\": \"execute_result\"\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"import re\\n\",\r\n", " \"my_string = \\\"this is a dog\\\"\\n\",\r\n", " \"re.sub(\\\"this\\\",\\\"That\\\",my_string)\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 150,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"data\": {\r\n", " \"text/plain\": [\r\n", " \"7\"\r\n", " ]\r\n", " },\r\n", " \"execution_count\": 150,\r\n", " \"metadata\": {},\r\n", " \"output_type\": \"execute_result\"\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"import random\\n\",\r\n", " \"random.randint(1, 10)\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"## Importing Modules\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"Excerpt from [Real Python post](https://realpython.com/python-modules-packages/)\\n\",\r\n", " \"\\n\",\r\n", " \"Modular programming refers to the process of breaking a large, unwieldy programming task into separate, smaller, more manageable subtasks or modules. Individual modules can then be cobbled together like building blocks to create a larger application.\\n\",\r\n", " \"\\n\",\r\n", " \"There are several advantages to modularizing code in a large application:\\n\",\r\n", " \"\\n\",\r\n", " \"- **Simplicity**: Rather than focusing on the entire problem at hand, a module typically focuses on one relatively small portion of the problem. If you’re working on a single module, you’ll have a smaller problem domain to wrap your head around. This makes development easier and less error-prone.\\n\",\r\n", " \"\\n\",\r\n", " \"- **Maintainability**: Modules are typically designed so that they enforce logical boundaries between different problem domains. If modules are written in a way that minimizes interdependency, there is decreased likelihood that modifications to a single module will have an impact on other parts of the program. (You may even be able to make changes to a module without having any knowledge of the application outside that module.) This makes it more viable for a team of many programmers to work collaboratively on a large application.\\n\",\r\n", " \"\\n\",\r\n", " \"- **Reusability**: Functionality defined in a single module can be easily reused (through an appropriately defined interface) by other parts of the application. This eliminates the need to recreate duplicate code.\\n\",\r\n", " \"\\n\",\r\n", " \"- **Scoping**: Modules typically define a separate namespace, which helps avoid collisions between identifiers in different areas of a program. (One of the tenets in the Zen of Python is Namespaces are one honking great idea—let’s do more of those!)\\n\",\r\n", " \"\\n\",\r\n", " \"Functions, modules and packages are all constructs in Python that promote code modularization.\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 8,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"import sys\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 5,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"import numpy as np\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 7,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"from sklearn import metrics\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"markdown\",\r\n", " \"metadata\": {},\r\n", " \"source\": [\r\n", " \"### Installing Modules\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 9,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"Requirement already satisfied: numpy in /Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/site-packages (1.15.1)\\n\",\r\n", " \"\\u001b[33mYou are using pip version 18.0, however version 19.2.3 is available.\\n\",\r\n", " \"You should consider upgrading via the 'pip install --upgrade pip' command.\\u001b[0m\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"!pip install numpy\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": 10,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [\r\n", " {\r\n", " \"name\": \"stdout\",\r\n", " \"output_type\": \"stream\",\r\n", " \"text\": [\r\n", " \"/bin/sh: conda: command not found\\r\\n\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"source\": [\r\n", " \"!conda install numpy\"\r\n", " ]\r\n", " },\r\n", " {\r\n", " \"cell_type\": \"code\",\r\n", " \"execution_count\": null,\r\n", " \"metadata\": {},\r\n", " \"outputs\": [],\r\n", " \"source\": [\r\n", " \"!\"\r\n", " ]\r\n", " }\r\n", " ],\r\n", " \"metadata\": {\r\n", " \"kernelspec\": {\r\n", " \"display_name\": \"Python 3\",\r\n", " \"language\": \"python\",\r\n", " \"name\": \"python3\"\r\n", " },\r\n", " \"language_info\": {\r\n", " \"codemirror_mode\": {\r\n", " \"name\": \"ipython\",\r\n", " \"version\": 3\r\n", " },\r\n", " \"file_extension\": \".py\",\r\n", " \"mimetype\": \"text/x-python\",\r\n", " \"name\": \"python\",\r\n", " \"nbconvert_exporter\": \"python\",\r\n", " \"pygments_lexer\": \"ipython3\",\r\n", " \"version\": \"3.7.0\"\r\n", " },\r\n", " \"toc\": {\r\n", " \"base_numbering\": 1,\r\n", " \"nav_menu\": {\r\n", " \"height\": \"495px\",\r\n", " \"width\": \"373.991px\"\r\n", " },\r\n", " \"number_sections\": false,\r\n", " \"sideBar\": true,\r\n", " \"skip_h1_title\": false,\r\n", " \"title_cell\": \"Table of Contents\",\r\n", " \"title_sidebar\": \"Contents\",\r\n", " \"toc_cell\": false,\r\n", " \"toc_position\": {\r\n", " \"height\": \"calc(100% - 180px)\",\r\n", " \"left\": \"10px\",\r\n", " \"top\": \"150px\",\r\n", " \"width\": \"165px\"\r\n", " },\r\n", " \"toc_section_display\": true,\r\n", " \"toc_window_display\": false\r\n", " }\r\n", " },\r\n", " \"nbformat\": 4,\r\n", " \"nbformat_minor\": 2\r\n", "}\r\n" ] } ], "source": [ "!cat lecture_03-using-jupyter-notebooks.ipynb" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# Initializing a Notebook\n", "\n", "There are two primary methods for initializing a notebook. \n", "\n", "1. **Via the command line**\n", " - Go into the working directory containing your `.ipynb` notebook. \n", " - e.g. `cd /Users/me/Desktop/`\n", " - type `jupyter notebook`\n", " - the web application will open up in your default browser. \n", " - from there, click on the notebook and \"spin it up\". The notebook will then be \"running\". \n", " - We can close the notebook by clicking on the `Quit` and `Logout` buttons on the page. \n", " - `Quit` == close the local server (i.e. the web application connection)\n", " - `Logout` == shut down the home page of the web application (but keep the server running)\n", " - We can also close the server connection in the console using the combo of `Control-C` in the console. \n", " - We can also relocate the the server (say if we accidentally close the Notebook) by using the local URL pathway provided when the notebook first activates.\n", "\n", "\n", "2. **Via the Anaconda Navigator** (requires you installing an [Anaconda distribution](https://www.anaconda.com/distribution/))\n", "\n", " - Click on the Anaconda Icon\n", " - Click \"Launch\" on the jupyter notebook icon.\n", " - The web application will immediately fire up (also yielding a console panel much like what we say via the command line approach). \n", " - One issue is that your working directory (i.e. where the notebook thinks you are on your computer) will be where ever Anaconda is stored (for me, it's at the very top of my file directory). Housing your projects here can be suboptimal for a whole range of reasons, so we'll need to **_change the working directory_** to the actual location that we want. \n", " - One benefit of spinning up a Jupyter notebook via the command line is that your working directory will always be where you initialized the notebook. " ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "---\n", "\n", "# Kernels\n", "\n", "A kernel is a computational engine that executes the code contained in a notebook document. A cell (or \"Chunk\") is a container for text to be displayed in the notebook or code to be executed by the notebook's kernel.\n", "\n", "Though we can only have one type of kernel running for any given notebook (we can't change between kernels in the middle of a notebook), we can use jupyter beyond just a python kernel. Here is a [list of all the kernels](https://github.com/jupyter/jupyter/wiki/Jupyter-kernels) that you can use with a jupyter notebook. For example, we can easily employ an [R kernel in a jupyter notebook](https://irkernel.github.io/). This was always the notebooks original intent. Actually, \"Jupyter\" is a loose acronym meaning Julia, Python and R" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "---\n", "\n", "# Usage\n", "\n", "\n", "## Code Chunks\n", "\n", "Code chunks are what we use to execute Python (or whatever kernel we have running) code. In addition, we can write prose in a code chunk by altering the metadata regarding how the code should be run.\n", "\n", "There are **two states** of a code chunk:\n", "\n", "- **Edit Mode**: Edit mode is indicated by a <font color =\"green\">green cell border</font> and a prompt showing in the editor area. When a cell is in edit mode, you can type into the cell, like a normal text editor. Enter edit mode by pressing Enter or using the mouse to click on a cell's editor area.\n", "\n", "\n", "- **Command Mode**: Command mode is indicated by a grey cell border with a <font color = \"blue\">blue left margin</font>. When you are in command mode, you are able to edit the notebook as a whole, but not type into individual cells. Most importantly, in command mode, the keyboard is mapped to a set of shortcuts that let you perform notebook and cell actions efficiently. For example, if you are in command mode and you press c, you will copy the current cell - no modifier is needed. Don't try to type into a cell in command mode. Enter command mode by pressing `Esc` or using the mouse to click outside a cell's editor area.\n", "\n", "We can **switch between Markdown and Code chunks** either \n", "\n", "- By using the **_drop down menu_** in the tool bar (in either mode)\n", "\n", "\n", "- By using the **_shortcut_**:\n", " - Press `y` when on the cell in Command Mode to switch to a code chunk.\n", " - Press `m` when on the cell in Command Mode to switch to a markdown chunk\n", "\n", "## Executing Code \n", "A code chunk will always reflect the behavior of the kernel that you're using (e.g. a Python code chunk will follow Python coding Syntax). \n", "\n", "**Best Practices**\n", "\n", "- Break code chunks up! \n", "- Every code chunk should render some output (the aim is to be able to read what we were doing without needing to fire the notebook back up)\n", "- Use spaces. Keep the chunk readable. Less is more.\n", "\n", "## Using Markdown\n", "The Markdown chunks will use the [Markdown](https://www.markdownguide.org/) and will allow for writing mathematical equations using LaTex. " ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# Header 1\n", "## Header 2\n", "### Header 3\n", "#### Header 4\n", "\n", "Bullet points \n", "\n", "- _Italics_ or *Italics*\n", "- **Bold**\n", "- **_Both_**\n", "\n", "Enumerated Lists\n", " \n", "1. _Italics_ or *Italics*\n", "2. **Bold**\n", "3. **_Both_**\n", "\n", "We can <u>underline</u> using html tags. Likewise, we can change <font color =\"darkred\">font colors</font>,\n", "\n", "<center> center </center>\n", "\n", "And include [hyperlinks](https://en.wikipedia.org/wiki/Grape)\n", "\n", "Write math inline with `$$`. For example, $y_i = \\beta_0 + \\beta_1 x_i + \\epsilon$\n", "\n", "Or stand alone,\n", "\n", "$$pr(y_i=1) = \\frac{1}{1+e^{\\beta_0 + \\beta_1 x_i + \\epsilon}}$$\n", "\n", "We can embed images. \n", "\n", "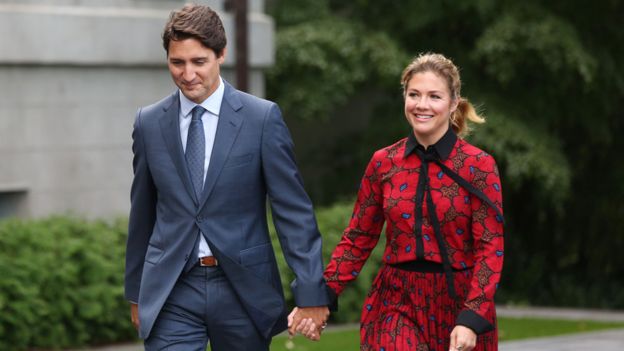\n", "\n", "And videos!" ] }, { "cell_type": "code", "execution_count": 2, "metadata": { "scrolled": true }, "outputs": [ { "data": { "text/html": [ "<iframe width=\"900\" height=\"300\" frameborder=\"0\" src=\"https://www.bbc.com/news/av/embed/p07n10lt/49656611\"></iframe>" ], "text/plain": [ "<IPython.core.display.HTML object>" ] }, "metadata": {}, "output_type": "display_data" } ], "source": [ "%%HTML\n", "<iframe width=\"900\" height=\"300\" frameborder=\"0\" src=\"https://www.bbc.com/news/av/embed/p07n10lt/49656611\"></iframe>" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Using the Shell (Command Line)\n", "\n", "As we saw in lab, we can use the command line from directly inside a notebook by preceding all shell code with a `!`. This allows use to really streamline our coding process. " ] }, { "cell_type": "code", "execution_count": 3, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "/Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03\r\n" ] } ], "source": [ "!pwd # Current working directory" ] }, { "cell_type": "code", "execution_count": 4, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "On branch master\r\n", "Your branch is up to date with 'origin/master'.\r\n", "\r\n", "Changes not staged for commit:\r\n", " (use \"git add <file>...\" to update what will be committed)\r\n", " (use \"git checkout -- <file>...\" to discard changes in working directory)\r\n", "\r\n", "\t\u001b[31mmodified: ../lecture_02/lecture_02_version-control.ipynb\u001b[m\r\n", "\r\n", "Untracked files:\r\n", " (use \"git add <file>...\" to include in what will be committed)\r\n", "\r\n", "\t\u001b[31m./\u001b[m\r\n", "\r\n", "no changes added to commit (use \"git add\" and/or \"git commit -a\")\r\n" ] } ], "source": [ "!git status #checking our git status (anything we need to commit?)" ] }, { "cell_type": "code", "execution_count": 5, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "lecture_03-using-jupyter-notebooks.ipynb\r\n", "my_fib_func.py\r\n" ] } ], "source": [ "!ls #list off all the files in the current working directory." ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "Also note that we can use inline magic to use shell commands." ] }, { "cell_type": "code", "execution_count": 6, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "1\n", "2\n", "3\n", "4\n", "5\n", "6\n", "7\n", "8\n", "9\n", "10\n" ] } ], "source": [ "%%sh \n", "for i in {1..10}\n", "do\n", " echo $i\n", "done" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Shortcuts\n", "\n", "As with most user interfaces, Jupyter Notebooks have developed their own way of doing things. Thus there are a number of useful shortcuts that you can employ to help perform useful tasks. \n", "\n", "We can access a full (searchable) list of keyboard shortcuts by pressing `p` when in Command Mode, or by clicking the keyboard icon in the tools.\n", "\n", "Important ones while in Command Mode:\n", "\n", "- `a`: create a new code chunk _above_ the current one.\n", "- `b`: create a new code chunk _below_ the current one.\n", "- `ii`: interrupt the kernel (really useful when some code is running too long or you've accidentally initiated an infinite loop!\n", "- `y`: code mode\n", "- `m`: markdown mode\n", "- `shift` + `m`: merge cells (when more than one cell is highlighted)\n", "\n", "Important ones while in Edit Mode:\n", "\n", "- `shit` + `ctrl` + `minus`: split cell" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "---\n", "\n", "# Magic Commands\n", "\n", "Magic commands, and are prefixed by the `%` character. These magic commands are designed to succinctly solve various common problems in standard data analysis. \n", "\n", "Magic commands come in two flavors: \n", "\n", "- **line magics**, which are denoted by a _single_ `%` prefix and operate on a single line of input, \n", "- **cell magics**, which are denoted by a _double_ `%%` prefix and operate on multiple lines of input. \n", "\n", "List off all the available magic commands." ] }, { "cell_type": "code", "execution_count": 7, "metadata": {}, "outputs": [ { "data": { "application/json": { "cell": { "!": "OSMagics", "HTML": "Other", "SVG": "Other", "bash": "Other", "capture": "ExecutionMagics", "debug": "ExecutionMagics", "file": "Other", "html": "DisplayMagics", "javascript": "DisplayMagics", "js": "DisplayMagics", "latex": "DisplayMagics", "markdown": "DisplayMagics", "perl": "Other", "prun": "ExecutionMagics", "pypy": "Other", "python2": "Other", "python3": "Other", "ruby": "Other", "script": "ScriptMagics", "sh": "Other", "svg": "DisplayMagics", "sx": "OSMagics", "system": "OSMagics", "time": "ExecutionMagics", "timeit": "ExecutionMagics", "writefile": "OSMagics" }, "line": { "alias": "OSMagics", "alias_magic": "BasicMagics", "autocall": "AutoMagics", "automagic": "AutoMagics", "autosave": "KernelMagics", "bookmark": "OSMagics", "cat": "Other", "cd": "OSMagics", "clear": "KernelMagics", "colors": "BasicMagics", "config": "ConfigMagics", "connect_info": "KernelMagics", "cp": "Other", "debug": "ExecutionMagics", "dhist": "OSMagics", "dirs": "OSMagics", "doctest_mode": "BasicMagics", "ed": "Other", "edit": "KernelMagics", "env": "OSMagics", "gui": "BasicMagics", "hist": "Other", "history": "HistoryMagics", "killbgscripts": "ScriptMagics", "ldir": "Other", "less": "KernelMagics", "lf": "Other", "lk": "Other", "ll": "Other", "load": "CodeMagics", "load_ext": "ExtensionMagics", "loadpy": "CodeMagics", "logoff": "LoggingMagics", "logon": "LoggingMagics", "logstart": "LoggingMagics", "logstate": "LoggingMagics", "logstop": "LoggingMagics", "ls": "Other", "lsmagic": "BasicMagics", "lx": "Other", "macro": "ExecutionMagics", "magic": "BasicMagics", "man": "KernelMagics", "matplotlib": "PylabMagics", "mkdir": "Other", "more": "KernelMagics", "mv": "Other", "notebook": "BasicMagics", "page": "BasicMagics", "pastebin": "CodeMagics", "pdb": "ExecutionMagics", "pdef": "NamespaceMagics", "pdoc": "NamespaceMagics", "pfile": "NamespaceMagics", "pinfo": "NamespaceMagics", "pinfo2": "NamespaceMagics", "pip": "BasicMagics", "popd": "OSMagics", "pprint": "BasicMagics", "precision": "BasicMagics", "profile": "BasicMagics", "prun": "ExecutionMagics", "psearch": "NamespaceMagics", "psource": "NamespaceMagics", "pushd": "OSMagics", "pwd": "OSMagics", "pycat": "OSMagics", "pylab": "PylabMagics", "qtconsole": "KernelMagics", "quickref": "BasicMagics", "recall": "HistoryMagics", "rehashx": "OSMagics", "reload_ext": "ExtensionMagics", "rep": "Other", "rerun": "HistoryMagics", "reset": "NamespaceMagics", "reset_selective": "NamespaceMagics", "rm": "Other", "rmdir": "Other", "run": "ExecutionMagics", "save": "CodeMagics", "sc": "OSMagics", "set_env": "OSMagics", "store": "StoreMagics", "sx": "OSMagics", "system": "OSMagics", "tb": "ExecutionMagics", "time": "ExecutionMagics", "timeit": "ExecutionMagics", "unalias": "OSMagics", "unload_ext": "ExtensionMagics", "who": "NamespaceMagics", "who_ls": "NamespaceMagics", "whos": "NamespaceMagics", "xdel": "NamespaceMagics", "xmode": "BasicMagics" } }, "text/plain": [ "Available line magics:\n", "%alias %alias_magic %autocall %automagic %autosave %bookmark %cat %cd %clear %colors %config %connect_info %cp %debug %dhist %dirs %doctest_mode %ed %edit %env %gui %hist %history %killbgscripts %ldir %less %lf %lk %ll %load %load_ext %loadpy %logoff %logon %logstart %logstate %logstop %ls %lsmagic %lx %macro %magic %man %matplotlib %mkdir %more %mv %notebook %page %pastebin %pdb %pdef %pdoc %pfile %pinfo %pinfo2 %popd %pprint %precision %profile %prun %psearch %psource %pushd %pwd %pycat %pylab %qtconsole %quickref %recall %rehashx %reload_ext %rep %rerun %reset %reset_selective %rm %rmdir %run %save %sc %set_env %store %sx %system %tb %time %timeit %unalias %unload_ext %who %who_ls %whos %xdel %xmode\n", "\n", "Available cell magics:\n", "%%! %%HTML %%SVG %%bash %%capture %%debug %%file %%html %%javascript %%js %%latex %%markdown %%perl %%prun %%pypy %%python %%python2 %%python3 %%ruby %%script %%sh %%svg %%sx %%system %%time %%timeit %%writefile\n", "\n", "Automagic is ON, % prefix IS NOT needed for line magics." ] }, "execution_count": 7, "metadata": {}, "output_type": "execute_result" } ], "source": [ "%lsmagic" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "Or consult the quick reference sheet of all available magic" ] }, { "cell_type": "code", "execution_count": 8, "metadata": {}, "outputs": [], "source": [ "%quickref" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Useful Magic\n", "\n", "Here are some useful magic commands that come in handy as you're working with code." ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Bookmarking\n", "\"Come back here later\"" ] }, { "cell_type": "code", "execution_count": 9, "metadata": {}, "outputs": [], "source": [ "%bookmark Home" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "See below" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Changing working directories " ] }, { "cell_type": "code", "execution_count": 10, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "/Users/ericdunford/Desktop\n" ] } ], "source": [ "%cd ~/Desktop" ] }, { "cell_type": "code", "execution_count": 11, "metadata": {}, "outputs": [ { "data": { "text/plain": [ "'/Users/ericdunford/Desktop'" ] }, "execution_count": 11, "metadata": {}, "output_type": "execute_result" } ], "source": [ "%pwd" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "Using the bookmark to return to where we were..." ] }, { "cell_type": "code", "execution_count": 12, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "(bookmark:Home) -> /Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03\n", "/Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03\n" ] } ], "source": [ "%cd -b Home" ] }, { "cell_type": "code", "execution_count": 13, "metadata": {}, "outputs": [ { "data": { "text/plain": [ "'/Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03'" ] }, "execution_count": 13, "metadata": {}, "output_type": "execute_result" } ], "source": [ "%pwd" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Writing code to files\n", "\n", "Extremely useful when we develop some functionality that we'd like to utilize later on." ] }, { "cell_type": "code", "execution_count": 14, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "Overwriting my_fib_func.py\n" ] } ], "source": [ "%%writefile my_fib_func.py\n", "def fib(n):\n", " '''Fibonacci Sequence'''\n", " x = [0]*n\n", " for i in range(n):\n", " if i == 0:\n", " x[i] = 0\n", " elif i == 1:\n", " x[i] = 1\n", " else:\n", " x[i] = x[i-2] + x[i-1]\n", " return x" ] }, { "cell_type": "code", "execution_count": 15, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "lecture_03-using-jupyter-notebooks.ipynb\r\n", "my_fib_func.py\r\n" ] } ], "source": [ "%ls # list files ( see our function)" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Reading in files" ] }, { "cell_type": "code", "execution_count": null, "metadata": {}, "outputs": [], "source": [ "# %load my_fib_func.py\n", "def fib(n):\n", " '''Fibonacci Sequence'''\n", " x = [0]*n\n", " for i in range(n):\n", " if i == 0:\n", " x[i] = 0\n", " elif i == 1:\n", " x[i] = 1\n", " else:\n", " x[i] = x[i-2] + x[i-1]\n", " return x" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Run an external file as a program" ] }, { "cell_type": "code", "execution_count": 17, "metadata": {}, "outputs": [], "source": [ "%run my_fib_func.py" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Timing Code\n", "\n", "How fast does what we wrote run?" ] }, { "cell_type": "code", "execution_count": 18, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "CPU times: user 3 µs, sys: 0 ns, total: 3 µs\n", "Wall time: 6.2 µs\n" ] }, { "data": { "text/plain": [ "[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]" ] }, "execution_count": 18, "metadata": {}, "output_type": "execute_result" } ], "source": [ "%time \n", "fib(10)" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "How long does many runs take (statistical sample)?" ] }, { "cell_type": "code", "execution_count": 19, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "2.98 µs ± 92.2 ns per loop (mean ± std. dev. of 7 runs, 100000 loops each)\n" ] } ], "source": [ "%%timeit\n", "fib(10)" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Look up object names in the name space" ] }, { "cell_type": "code", "execution_count": 20, "metadata": {}, "outputs": [], "source": [ "main_dat = [1,2,3,4]\n", "main_key = [\"a\",\"b\"]\n", "x = 5\n", "y = 6" ] }, { "cell_type": "code", "execution_count": 21, "metadata": {}, "outputs": [], "source": [ "%psearch main*" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "Whenever you encounter an error or exception, just open a new notebook cell, type `%debug` and run the cell. This will open a command line where you can test your code and inspect all variables right up to the line that threw the error. Type `n` and hit Enter to run the next line of code (The `->` arrow shows you the current position). Use `c` to continue until the next breakpoint. `q` quits the debugger and code execution." ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Asking for help" ] }, { "cell_type": "code", "execution_count": 22, "metadata": {}, "outputs": [], "source": [ "%%timeit?" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "---\n", "\n", "# Notebook Extensions\n", "\n", "We can expand the functionality of Jupyter notebooks through extensions. Extensions allow for use to create and use new features that better customize the notebook's user experience. For example, there are extensions for spell check, a table of contents to ease navigation, run code in parallel, and for viewing differences in notebooks when using Version control.\n", "\n", "Download python module to install notebook extensions: https://github.com/ipython-contrib/jupyter_contrib_nbextensions\n", "\n", "\n", "Using `PyPi` (module manager):\n", "```\n", "pip install jupyter_nbextensions_configurator jupyter_contrib_nbextensions\n", "jupyter contrib nbextension install --user\n", "jupyter nbextensions_configurator enable --user\n", "```\n", "\n", "Using `Conda` (Anaconda module manager):\n", "```\n", "conda install -c conda-forge jupyter_contrib_nbextensions\n", "jupyter contrib nbextension install --user\n", "jupyter nbextensions_configurator enable --user\n", "```\n", "\n", "Extensions can be activated most easily on the home screen when you first activate your Jupyter notebook.\n", "\n", "\n", "## Useful Extensions\n", "\n", "- **Collapsible headings**: allows you to collapse some parts of the notebooks.\n", "- **Notify**: sends a notification when the notebook becomes idle (for long running tasks)\n", "- **Code folding**: folds function, loops, and indented code chunks (makes things tidy)\n", "- **nbdime**: provides tools for git differencing and merging of Jupyter Notebooks.\n", " - Requires installation: `pip install nbdime`" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "---\n", "\n", "# Being Pythonic\n", "\n", "- whitespace is significant\n", " - Indentations demarcate code blocks. \n", " - Four spaces == indentation (PEP8)\n", "- everything is an object\n", "- the aim is readable code\n", "- Updates to python are recorded in [Python Enhancement Proposals](https://www.python.org/dev/peps/) (or PEPs)\n", " - When there is a change to python, it is recorded here\n", " - also the python \"philosophy\" lives here in its suggestions (e.g. PEP8 re: spacing)" ] }, { "cell_type": "code", "execution_count": 23, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "The Zen of Python, by Tim Peters\n", "\n", "Beautiful is better than ugly.\n", "Explicit is better than implicit.\n", "Simple is better than complex.\n", "Complex is better than complicated.\n", "Flat is better than nested.\n", "Sparse is better than dense.\n", "Readability counts.\n", "Special cases aren't special enough to break the rules.\n", "Although practicality beats purity.\n", "Errors should never pass silently.\n", "Unless explicitly silenced.\n", "In the face of ambiguity, refuse the temptation to guess.\n", "There should be one-- and preferably only one --obvious way to do it.\n", "Although that way may not be obvious at first unless you're Dutch.\n", "Now is better than never.\n", "Although never is often better than *right* now.\n", "If the implementation is hard to explain, it's a bad idea.\n", "If the implementation is easy to explain, it may be a good idea.\n", "Namespaces are one honking great idea -- let's do more of those!\n" ] } ], "source": [ "# Python has a sort of philosophy to it. \n", "import this " ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Standard Library\n", "\n", "Python comes with an extensive [standard library](https://docs.python.org/3/library/) and built-in functions." ] }, { "cell_type": "code", "execution_count": 24, "metadata": {}, "outputs": [ { "data": { "text/plain": [ "4.605170185988092" ] }, "execution_count": 24, "metadata": {}, "output_type": "execute_result" } ], "source": [ "import math\n", "math.log(100)" ] }, { "cell_type": "code", "execution_count": 25, "metadata": {}, "outputs": [ { "data": { "text/plain": [ "'That is a dog'" ] }, "execution_count": 25, "metadata": {}, "output_type": "execute_result" } ], "source": [ "import re\n", "my_string = \"this is a dog\"\n", "re.sub(\"this\",\"That\",my_string)" ] }, { "cell_type": "code", "execution_count": 26, "metadata": {}, "outputs": [ { "data": { "text/plain": [ "10" ] }, "execution_count": 26, "metadata": {}, "output_type": "execute_result" } ], "source": [ "import random\n", "random.randint(1, 10)" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Importing Modules" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "Excerpt from [Real Python post](https://realpython.com/python-modules-packages/)\n", "\n", "Modular programming refers to the process of breaking a large, unwieldy programming task into separate, smaller, more manageable subtasks or modules. Individual modules can then be cobbled together like building blocks to create a larger application.\n", "\n", "There are several advantages to modularizing code in a large application:\n", "\n", "- **Simplicity**: Rather than focusing on the entire problem at hand, a module typically focuses on one relatively small portion of the problem. If you’re working on a single module, you’ll have a smaller problem domain to wrap your head around. This makes development easier and less error-prone.\n", "\n", "- **Maintainability**: Modules are typically designed so that they enforce logical boundaries between different problem domains. If modules are written in a way that minimizes interdependency, there is decreased likelihood that modifications to a single module will have an impact on other parts of the program. (You may even be able to make changes to a module without having any knowledge of the application outside that module.) This makes it more viable for a team of many programmers to work collaboratively on a large application.\n", "\n", "- **Reusability**: Functionality defined in a single module can be easily reused (through an appropriately defined interface) by other parts of the application. This eliminates the need to recreate duplicate code.\n", "\n", "- **Scoping**: Modules typically define a separate namespace, which helps avoid collisions between identifiers in different areas of a program. (One of the tenets in the Zen of Python is Namespaces are one honking great idea—let’s do more of those!)\n", "\n", "Functions, modules and packages are all constructs in Python that promote code modularization." ] }, { "cell_type": "code", "execution_count": 27, "metadata": {}, "outputs": [], "source": [ "import sys" ] }, { "cell_type": "code", "execution_count": 28, "metadata": {}, "outputs": [], "source": [ "import numpy as np" ] }, { "cell_type": "code", "execution_count": 29, "metadata": {}, "outputs": [], "source": [ "from sklearn import metrics" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Installing Modules" ] }, { "cell_type": "code", "execution_count": 30, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "Requirement already satisfied: numpy in /Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/site-packages (1.15.1)\n", "\u001b[33mYou are using pip version 18.0, however version 19.2.3 is available.\n", "You should consider upgrading via the 'pip install --upgrade pip' command.\u001b[0m\n" ] } ], "source": [ "!pip install numpy" ] }, { "cell_type": "code", "execution_count": 31, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "/bin/sh: conda: command not found\r\n" ] } ], "source": [ "!conda install numpy" ] } ], "metadata": { "kernelspec": { "display_name": "Python 3", "language": "python", "name": "python3" }, "language_info": { "codemirror_mode": { "name": "ipython", "version": 3 }, "file_extension": ".py", "mimetype": "text/x-python", "name": "python", "nbconvert_exporter": "python", "pygments_lexer": "ipython3", "version": "3.7.2" }, "toc": { "base_numbering": 1, "nav_menu": { "height": "495px", "width": "373.991px" }, "number_sections": false, "sideBar": true, "skip_h1_title": false, "title_cell": "Table of Contents", "title_sidebar": "Contents", "toc_cell": false, "toc_position": { "height": "calc(100% - 180px)", "left": "10px", "top": "150px", "width": "165px" }, "toc_section_display": true, "toc_window_display": false } }, "nbformat": 4, "nbformat_minor": 2 }
There are two primary methods for initializing a notebook.
.ipynb
notebook. cd /Users/me/Desktop/
jupyter notebook
Quit
and Logout
buttons on the page. Quit
== close the local server (i.e. the web application connection)Logout
== shut down the home page of the web application (but keep the server running)Control-C
in the console. Via the Anaconda Navigator (requires you installing an Anaconda distribution)
A kernel is a computational engine that executes the code contained in a notebook document. A cell (or "Chunk") is a container for text to be displayed in the notebook or code to be executed by the notebook's kernel.
Though we can only have one type of kernel running for any given notebook (we can't change between kernels in the middle of a notebook), we can use jupyter beyond just a python kernel. Here is a list of all the kernels that you can use with a jupyter notebook. For example, we can easily employ an R kernel in a jupyter notebook. This was always the notebooks original intent. Actually, "Jupyter" is a loose acronym meaning Julia, Python and R
Code chunks are what we use to execute Python (or whatever kernel we have running) code. In addition, we can write prose in a code chunk by altering the metadata regarding how the code should be run.
There are two states of a code chunk:
Esc
or using the mouse to click outside a cell's editor area.We can switch between Markdown and Code chunks either
y
when on the cell in Command Mode to switch to a code chunk.m
when on the cell in Command Mode to switch to a markdown chunkA code chunk will always reflect the behavior of the kernel that you're using (e.g. a Python code chunk will follow Python coding Syntax).
Best Practices
The Markdown chunks will use the Markdown and will allow for writing mathematical equations using LaTex.
Bullet points
Enumerated Lists
We can underline using html tags. Likewise, we can change <font color ="darkred">font colors</font>,
And include hyperlinks
Write math inline with $$
. For example, $y_i = \beta_0 + \beta_1 x_i + \epsilon$
Or stand alone,
$$pr(y_i=1) = \frac{1}{1+e^{\beta_0 + \beta_1 x_i + \epsilon}}$$
We can embed images.
And videos!
%%HTML
<iframe width="900" height="300" frameborder="0" src="https://www.bbc.com/news/av/embed/p07n10lt/49656611"></iframe>
As we saw in lab, we can use the command line from directly inside a notebook by preceding all shell code with a !
. This allows use to really streamline our coding process.
!pwd # Current working directory
/Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03
!git status #checking our git status (anything we need to commit?)
On branch master Your branch is up to date with 'origin/master'. Changes not staged for commit: (use "git add <file>..." to update what will be committed) (use "git checkout -- <file>..." to discard changes in working directory) modified: ../lecture_02/lecture_02_version-control.ipynb Untracked files: (use "git add <file>..." to include in what will be committed) ./ no changes added to commit (use "git add" and/or "git commit -a")
!ls #list off all the files in the current working directory.
lecture_03-using-jupyter-notebooks.ipynb my_fib_func.py
Also note that we can use inline magic to use shell commands.
%%sh
for i in {1..10}
do
echo $i
done
1 2 3 4 5 6 7 8 9 10
As with most user interfaces, Jupyter Notebooks have developed their own way of doing things. Thus there are a number of useful shortcuts that you can employ to help perform useful tasks.
We can access a full (searchable) list of keyboard shortcuts by pressing p
when in Command Mode, or by clicking the keyboard icon in the tools.
Important ones while in Command Mode:
a
: create a new code chunk above the current one.b
: create a new code chunk below the current one.ii
: interrupt the kernel (really useful when some code is running too long or you've accidentally initiated an infinite loop!y
: code modem
: markdown modeshift
+ m
: merge cells (when more than one cell is highlighted)Important ones while in Edit Mode:
shit
+ ctrl
+ minus
: split cellMagic commands, and are prefixed by the %
character. These magic commands are designed to succinctly solve various common problems in standard data analysis.
Magic commands come in two flavors:
%
prefix and operate on a single line of input, %%
prefix and operate on multiple lines of input. List off all the available magic commands.
%lsmagic
Available line magics: %alias %alias_magic %autocall %automagic %autosave %bookmark %cat %cd %clear %colors %config %connect_info %cp %debug %dhist %dirs %doctest_mode %ed %edit %env %gui %hist %history %killbgscripts %ldir %less %lf %lk %ll %load %load_ext %loadpy %logoff %logon %logstart %logstate %logstop %ls %lsmagic %lx %macro %magic %man %matplotlib %mkdir %more %mv %notebook %page %pastebin %pdb %pdef %pdoc %pfile %pinfo %pinfo2 %popd %pprint %precision %profile %prun %psearch %psource %pushd %pwd %pycat %pylab %qtconsole %quickref %recall %rehashx %reload_ext %rep %rerun %reset %reset_selective %rm %rmdir %run %save %sc %set_env %store %sx %system %tb %time %timeit %unalias %unload_ext %who %who_ls %whos %xdel %xmode Available cell magics: %%! %%HTML %%SVG %%bash %%capture %%debug %%file %%html %%javascript %%js %%latex %%markdown %%perl %%prun %%pypy %%python %%python2 %%python3 %%ruby %%script %%sh %%svg %%sx %%system %%time %%timeit %%writefile Automagic is ON, % prefix IS NOT needed for line magics.
Or consult the quick reference sheet of all available magic
%quickref
Here are some useful magic commands that come in handy as you're working with code.
"Come back here later"
%bookmark Home
See below
%cd ~/Desktop
/Users/edunford/Desktop
%pwd
'/Users/edunford/Desktop'
Using the bookmark to return to where we were...
%cd -b Home
UsageError: Bookmark 'Home' not found. Use '%bookmark -l' to see your bookmarks.
%pwd
'/Users/ericdunford/Dropbox/Georgetown/Courses/PPOL564-Foundations/lectures/lecture_03'
Extremely useful when we develop some functionality that we'd like to utilize later on.
%%writefile my_fib_func.py
def fib(n):
'''Fibonacci Sequence'''
x = [0]*n
for i in range(n):
if i == 0:
x[i] = 0
elif i == 1:
x[i] = 1
else:
x[i] = x[i-2] + x[i-1]
return x
Writing my_fib_func.py
%ls # list files ( see our function)
lecture_03-using-jupyter-notebooks.ipynb my_fib_func.py
# %load my_fib_func.py
def fib(n):
'''Fibonacci Sequence'''
x = [0]*n
for i in range(n):
if i == 0:
x[i] = 0
elif i == 1:
x[i] = 1
else:
x[i] = x[i-2] + x[i-1]
return x
%run my_fib_func.py
How fast does what we wrote run?
%time fib(10)
CPU times: user 12 µs, sys: 0 ns, total: 12 µs Wall time: 16.9 µs
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
How long does many runs take (statistical sample)?
%%timeit
fib(10)
2.66 µs ± 32.9 ns per loop (mean ± std. dev. of 7 runs, 100000 loops each)
main_dat = [1,2,3,4]
main_key = ["a","b"]
x = 5
y = 6
%psearch main*
Whenever you encounter an error or exception, just open a new notebook cell, type %debug
and run the cell. This will open a command line where you can test your code and inspect all variables right up to the line that threw the error. Type n
and hit Enter to run the next line of code (The ->
arrow shows you the current position). Use c
to continue until the next breakpoint. q
quits the debugger and code execution.
%%timeit?
We can expand the functionality of Jupyter notebooks through extensions. Extensions allow for use to create and use new features that better customize the notebook's user experience. For example, there are extensions for spell check, a table of contents to ease navigation, run code in parallel, and for viewing differences in notebooks when using Version control.
Download python module to install notebook extensions: https://github.com/ipython-contrib/jupyter_contrib_nbextensions
Using PyPi
(module manager):
pip install jupyter_nbextensions_configurator jupyter_contrib_nbextensions
jupyter contrib nbextension install --user
jupyter nbextensions_configurator enable --user
Using Conda
(Anaconda module manager):
conda install -c conda-forge jupyter_contrib_nbextensions
jupyter contrib nbextension install --user
jupyter nbextensions_configurator enable --user
Extensions can be activated most easily on the home screen when you first activate your Jupyter notebook.
pip install nbdime